基于 Python,利用蒙特卡洛模拟方法,探索解决一个蒙特卡洛模拟在金融领域的应用实例
时间: 2024-03-19 19:43:51 浏览: 22
一个经典的蒙特卡洛模拟在金融领域的应用实例是模拟股票价格的波动。下面是一个简单的示例代码,用于模拟股票价格的波动,并计算在不同情况下的投资回报率。
```python
import numpy as np
import matplotlib.pyplot as plt
# 股票价格的初始值和波动率
initial_price = 100
volatility = 0.2
# 模拟的时间段和时间步长
time_period = 252
time_step = 1
# 模拟的次数
num_simulations = 1000
# 生成随机数
random_numbers = np.random.normal(0, 1, (time_period, num_simulations))
# 生成股票价格的路径
prices = np.zeros((time_period, num_simulations))
prices[0] = initial_price
for t in range(1, time_period):
prices[t] = prices[t-1] * np.exp((0.5 * volatility ** 2 - 1) * time_step + volatility * np.sqrt(time_step) * random_numbers[t])
# 计算投资回报率
returns = np.zeros(num_simulations)
for i in range(num_simulations):
returns[i] = (prices[-1, i] - initial_price) / initial_price
# 绘制股票价格路径
plt.plot(prices)
plt.title('Stock Price Simulation')
plt.xlabel('Time')
plt.ylabel('Price')
plt.show()
# 绘制投资回报率的直方图
plt.hist(returns, bins=50)
plt.title('Investment Returns')
plt.xlabel('Return')
plt.ylabel('Frequency')
plt.show()
# 计算投资回报率的均值和标准差
mean_return = np.mean(returns)
std_return = np.std(returns)
# 打印投资回报率的统计信息
print('Mean return: {:.2f}%'.format(mean_return * 100))
print('Standard deviation of return: {:.2f}%'.format(std_return * 100))
```
在上述代码中,我们使用了 NumPy 库来生成随机数,并使用了 matplotlib 库来绘制股票价格路径和投资回报率的直方图。代码中使用了蒙特卡洛模拟方法,通过生成随机数来模拟股票价格的波动,并计算在不同情况下的投资回报率。最后,我们计算了投资回报率的均值和标准差,并打印出统计信息。
需要注意的是,上述代码只是一个简单的示例,实际应用中需要考虑更多的因素,例如股票价格的趋势、股票的基本面分析等。同时,蒙特卡洛模拟也有一定的局限性,例如需要考虑数据的随机性、模型的准确性等问题。
相关推荐
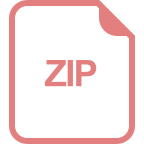
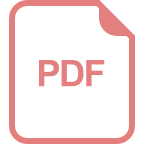














