ESP32天气预报时钟连接WIFI后如何获取天气预报和时间等数据
时间: 2024-06-08 08:10:50 浏览: 190
要获取天气预报和时间等数据,您需要使用ESP32连接到互联网,并使用适当的API从互联网获取数据。以下是一些步骤:
1. 首先,您需要连接ESP32到WIFI网络。您可以使用ESP32的WIFI库来实现这一点。以下是一个简单的示例代码:
```
#include <WiFi.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
void loop() {
// Your code here
}
```
2. 接下来,您需要找到一个提供天气预报和时间数据的API。其中一些常用的API包括OpenWeatherMap和Weather Underground。您需要注册并获取API密钥才能使用这些API。
3. 然后,您需要使用ESP32的HTTPClient库从API中获取数据。以下是一个简单的示例代码:
```
#include <WiFi.h>
#include <HTTPClient.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* api_key = "your_API_KEY";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
HTTPClient http;
String url = "https://api.openweathermap.org/data/2.5/weather?q=London,uk&APPID=";
url += api_key;
http.begin(url);
int httpCode = http.GET();
if (httpCode > 0) {
String payload = http.getString();
Serial.println(payload);
}
http.end();
}
void loop() {
// Your code here
}
```
请注意,上面的代码将获取伦敦的天气预报数据。您需要将URL中的“London,uk”更改为您想要获取天气预报的城市。
4. 最后,您需要解析从API获取的JSON数据,并在ESP32上显示天气预报和时间等数据。这可能需要一些额外的编程,具体取决于您使用的API和您的显示设备。
希望这可以帮助您开始获取天气预报和时间等数据!
阅读全文
相关推荐
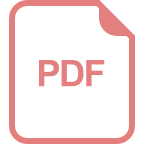
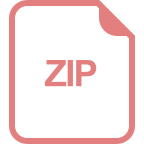
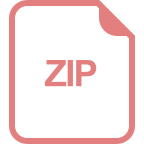
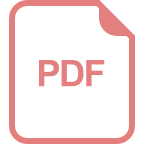
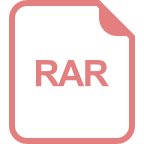
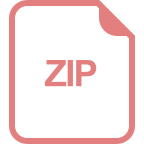
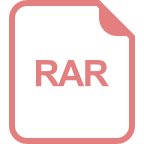
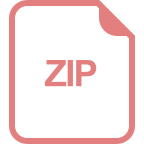
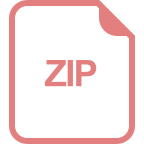
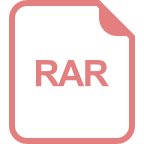
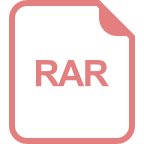
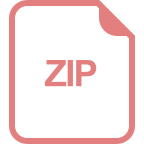
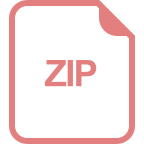
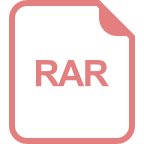