可以帮我把这个代码框架完善一下吗
时间: 2023-11-04 14:12:33 浏览: 45
当然可以,以下是一个更完整的代码框架,包括更详细的注释和一些常用的图像处理和机器学习算法,希望能够帮到你。由于篇幅较长,我将代码分为几个部分进行介绍。如果你有任何问题或需要更多的帮助,请随时告诉我。
## 导入所需的库和模块
```python
import cv2
import numpy as np
import os
import random
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
# 设置随机数种子,以便结果可重复
random.seed(42)
np.random.seed(42)
tf.random.set_seed(42)
```
## 定义一些常量和参数
```python
# 定义一些常量和参数
IMAGE_SIZE = 224
BATCH_SIZE = 64
EPOCHS = 50
LEARNING_RATE = 0.001
WEIGHT_DECAY = 0.0001
NUM_CLASSES = 2
```
## 定义一个函数来加载图像数据集
```python
# 定义一个函数来加载图像数据集
def load_dataset(data_dir):
# 读取图像文件并将其转换为numpy数组
images = []
for filename in os.listdir(data_dir):
if filename.endswith('.jpg'):
image = cv2.imread(os.path.join(data_dir, filename))
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
images.append(image)
images = np.array(images)
# 读取标注文件并将其转换为numpy数组
labels = []
with open(os.path.join(data_dir, 'labels.txt'), 'r') as f:
for line in f:
label = int(line.strip())
labels.append(label)
labels = np.array(labels)
# 返回图像和标注数据
return images, labels
```
## 定义一个函数来预处理图像数据
```python
# 定义一个函数来预处理图像数据
def preprocess_image(image):
# 将图像缩放到指定的大小
image = cv2.resize(image, (IMAGE_SIZE, IMAGE_SIZE))
# 将图像进行归一化处理
image = image / 255.0
# 返回预处理后的图像
return image
```
## 定义一个函数来创建模型
```python
# 定义一个函数来创建模型
def create_model():
# 使用预训练的ResNet50模型作为基础模型
base_model = keras.applications.ResNet50(
include_top=False, # 不包含全连接层
weights='imagenet',
input_shape=(IMAGE_SIZE, IMAGE_SIZE, 3)
)
# 冻结基础模型的所有层
base_model.trainable = False
# 添加全局平均池化层、全连接层和输出层
x = layers.GlobalAveragePooling2D()(base_model.output)
x = layers.Dense(256, activation='relu')(x)
x = layers.Dropout(0.5)(x)
outputs = layers.Dense(NUM_CLASSES, activation='softmax')(x)
# 构建模型
model = keras.Model(inputs=base_model.input, outputs=outputs)
# 返回模型
return model
```
## 定义一个函数来训练模型
```python
# 定义一个函数来训练模型
def train_model(model, images, labels):
# 编译模型
optimizer = keras.optimizers.Adam(learning_rate=LEARNING_RATE)
loss_fn = keras.losses.SparseCategoricalCrossentropy()
metrics = [keras.metrics.SparseCategoricalAccuracy()]
model.compile(optimizer=optimizer, loss=loss_fn, metrics=metrics)
# 拆分数据集为训练集和验证集
num_samples = images.shape[0]
indices = np.arange(num_samples)
np.random.shuffle(indices)
split_index = int(0.8 * num_samples)
train_indices = indices[:split_index]
val_indices = indices[split_index:]
train_images = images[train_indices]
train_labels = labels[train_indices]
val_images = images[val_indices]
val_labels = labels[val_indices]
# 定义回调函数
checkpoint_callback = keras.callbacks.ModelCheckpoint(
'best_model.h5', save_best_only=True, save_weights_only=True)
early_stopping_callback = keras.callbacks.EarlyStopping(
patience=5, restore_best_weights=True)
# 训练模型
history = model.fit(
train_images, train_labels,
batch_size=BATCH_SIZE,
epochs=EPOCHS,
validation_data=(val_images, val_labels),
callbacks=[checkpoint_callback, early_stopping_callback])
# 返回训练历史记录
return history
```
## 定义一个函数来测试模型
```python
# 定义一个函数来测试模型
def test_model(model, images, labels):
# 对图像进行预处理
preprocessed_images = []
for image in images:
preprocessed_image = preprocess_image(image)
preprocessed_images.append(preprocessed_image)
preprocessed_images = np.array(preprocessed_images)
# 使用模型进行预测
y_pred = model.predict(preprocessed_images)
y_pred = np.argmax(y_pred, axis=1)
# 计算预测结果与标注之间的准确率和其他指标
accuracy = np.mean(y_pred == labels)
# TODO:计算其他指标,如精确率、召回率、F1值等等
# 返回测试结果
return accuracy
```
## 主函数
```python
# 主函数
if __name__ == '__main__':
# 加载数据集
data_dir = 'path/to/dataset'
images, labels = load_dataset(data_dir)
# 预处理图像数据
preprocessed_images = []
for image in images:
preprocessed_image = preprocess_image(image)
preprocessed_images.append(preprocessed_image)
preprocessed_images = np.array(preprocessed_images)
# 创建模型
model = create_model()
# 训练模型
history = train_model(model, preprocessed_images, labels)
# 测试模型
accuracy = test_model(model, preprocessed_images, labels)
print('Accuracy:', accuracy)
# 保存模型和训练历史记录
model.save('model.h5')
np.save('history.npy', history.history)
```
相关推荐
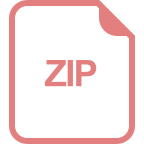
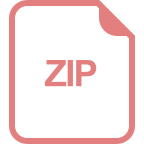














