use C program To delete a character that is the same as a character in a string, you need to use a character pointer as a function parameter. call the function in main. The function prototype is as follows: void delete_ character(char *str, char ch);
时间: 2024-03-18 18:39:31 浏览: 62
Sure, here's an implementation of the delete_character function in C:
```
#include <stdio.h>
void delete_character(char *str, char ch) {
int i, j;
for (i = 0, j = 0; str[i] != '\0'; i++) {
if (str[i] != ch) {
str[j] = str[i];
j++;
}
}
str[j] = '\0';
}
int main() {
char str[100], ch;
printf("Enter a string: ");
scanf("%s", str);
printf("Enter a character to delete: ");
scanf(" %c", &ch);
delete_character(str, ch);
printf("String after deleting '%c': %s\n", ch, str);
return 0;
}
```
The function takes two parameters: a pointer to the string to modify, and the character to delete. It loops through the string, copying each character to a new position in the string only if it's not equal to the character to delete. Finally, it adds a null terminator to the end of the new string to indicate its end.
In main, we get the string and character to delete from the user, call the delete_character function, and print the resulting string.
阅读全文
相关推荐
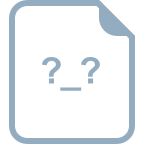
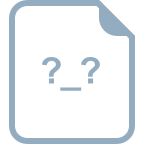

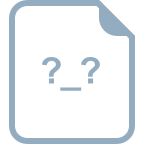
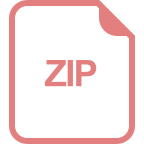
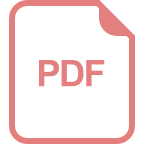
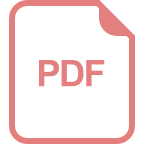
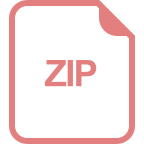
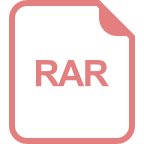
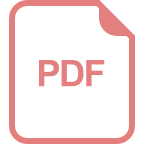
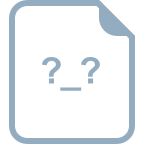
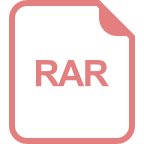