用户编写的shell脚本是否需要编译?shell脚本与C语言程序有什么区别
时间: 2024-02-21 19:25:32 浏览: 208
用户编写的shell脚本不需要编译,它们是解释型语言。当你运行shell脚本时,操作系统会读取脚本文件的内容,并将其解释成一系列可执行的命令来执行。
与C语言程序相比,shell脚本具有以下区别:
1. C程序需要先编译成二进制文件再执行,而shell脚本则可以直接运行,无需编译。
2. C程序通常需要显式地使用变量和函数来编写程序,而shell脚本则可以直接使用命令和管道来完成大多数任务。
3. C程序可以直接访问系统和硬件资源,而shell脚本则通常被限制在操作系统提供的命令和工具之内。
4. C程序通常需要一些编程经验和知识,而shell脚本则可以由非程序员编写和使用。
相关问题
C语言跟Java不能直接编写shell脚本吧?只能用模拟实现shell程序,这个过程中两者有什么区别
C语言和Java都不能直接编写shell脚本,因为它们是编译型语言,而shell脚本是解释型语言。不过,可以通过模拟实现shell程序的方式来使用C语言和Java编写类似shell的功能。在这个过程中,C语言和Java有以下区别:
1. **执行方式**:
- **C语言**:C语言编写的程序需要编译成可执行文件,然后通过终端或命令行执行。
- **Java**:Java编写的程序需要编译成字节码,然后通过Java虚拟机(JVM)执行。
2. **系统调用**:
- **C语言**:可以直接使用系统调用(如`fork()`, `exec()`, `pipe()`, `dup2()`等)来实现shell的功能。这些系统调用提供了对操作系统底层功能的直接访问。
- **Java**:Java通过`Runtime`类和`ProcessBuilder`类来执行系统命令。这些类封装了系统调用,提供了一个更高层次的接口。
3. **代码复杂度**:
- **C语言**:由于可以直接使用系统调用,C语言的代码通常更接近底层,复杂度较高,需要手动管理内存和处理系统资源。
- **Java**:Java提供了更高层次的抽象,代码通常更简洁,易于维护,但可能不如C语言高效。
4. **跨平台性**:
- **C语言**:C语言编写的程序需要在不同平台上重新编译,跨平台性较差。
- **Java**:Java编写的程序可以在任何安装了JVM的平台上运行,具有良好的跨平台性。
以下是一个简单的例子,展示如何在C语言和Java中模拟实现一个基本的shell命令执行功能:
**C语言示例**:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
char command[256];
while (1) {
printf("Enter command: ");
fgets(command, sizeof(command), stdin);
command[strcspn(command, "\n")] = 0; // Remove newline character
if (fork() == 0) {
execlp(command, command, NULL);
perror("execlp");
exit(1);
} else {
wait(NULL);
}
}
return 0;
}
```
**Java示例**:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class SimpleShell {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String command;
while (true) {
System.out.print("Enter command: ");
command = reader.readLine();
if (command == null || command.isEmpty()) {
continue;
}
Process process = Runtime.getRuntime().exec(command);
process.waitFor();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在Linux系统下编写c语言程序,在屏幕上输入10!的计算结果,使用shell脚本编写命令,在脚本中完成编译和执行
在Linux系统下,你可以通过创建一个C语言源文件,然后使用shell脚本来编译并执行该程序来完成这个任务。首先,假设你的C源文件名为`factorial.c`,内容如下:
```c
#include <stdio.h>
int factorial(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial(n - 1);
}
int main() {
int number = 10;
printf("10! 的计算结果是 %d\n", factorial(number));
return 0;
}
```
接下来,你需要创建一个shell脚本(例如`run_factorial.sh`),在其中编写编译和执行的命令:
```bash
#!/bin/bash
# 定义编译器路径,这里假设是gcc
CC=/usr/bin/gcc
# 编译源文件
if [ $? -eq 0 ]; then
# 使用makefile简化编译过程,如果没有makefile,可以手动编译:$CC factorial.c -o factorial
make factorial
else
echo "编译失败"
exit 1
fi
# 检查编译是否成功
if [ -f factorial ]; then
# 执行编译后的程序
./factorial
else
echo "无法找到编译后的程序"
exit 1
fi
```
保存脚本后,需要给它执行权限:
```bash
chmod +x run_factorial.sh
```
最后,运行shell脚本:
```bash
./run_factorial.sh
```
这将显示10的阶乘的结果。
阅读全文
相关推荐
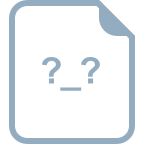















