帮我用javascript写一个烟花功能
时间: 2023-08-28 16:33:01 浏览: 174
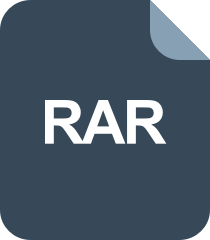
一个产生烟花的java程序
好的,下面是一个简单的烟花效果的JavaScript代码,你可以将其放置在HTML文件中:
```html
<canvas id="fireworks"></canvas>
```
```javascript
// 获取画布对象
const canvas = document.getElementById('fireworks');
const ctx = canvas.getContext('2d');
// 设置画布的大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 定义烟花的颜色
const colors = [
'#FF4136', '#0074D9', '#2ECC40', '#FFDC00',
'#FF851B', '#B10DC9', '#01FF70', '#F012BE'
];
// 定义烟花的粒子数
const particles = [];
// 定义烟花的构造函数
function Firework() {
this.x = Math.random() * canvas.width;
this.y = canvas.height;
this.color = colors[Math.floor(Math.random() * colors.length)];
this.radius = 4;
this.velocity = {
x: Math.random() * 6 - 3,
y: Math.random() * 3 + 2
};
this.gravity = 0.1;
}
// 更新烟花的位置
Firework.prototype.update = function() {
this.x += this.velocity.x;
this.y -= this.velocity.y;
this.velocity.y -= this.gravity;
};
// 绘制烟花
Firework.prototype.draw = function() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.fill();
};
// 创建烟花粒子
function createParticles(x, y) {
for (let i = 0; i < 30; i++) {
const particle = new Particle(x, y);
particles.push(particle);
}
}
// 定义烟花粒子的构造函数
function Particle(x, y) {
this.x = x;
this.y = y;
this.color = colors[Math.floor(Math.random() * colors.length)];
this.radius = Math.random() * 2;
this.velocity = {
x: Math.random() * 6 - 3,
y: Math.random() * 6 - 3
};
this.gravity = 0.1;
this.alpha = 1;
}
// 更新烟花粒子的位置
Particle.prototype.update = function() {
this.x += this.velocity.x;
this.y += this.velocity.y;
this.velocity.y += this.gravity;
this.alpha -= 0.01;
};
// 绘制烟花粒子
Particle.prototype.draw = function() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.globalAlpha = this.alpha;
ctx.fill();
};
// 渲染烟花效果
function render() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
if (Math.random() < 0.05) {
const firework = new Firework();
createParticles(firework.x, firework.y);
}
particles.forEach((particle, index) => {
particle.update();
particle.draw();
if (particle.alpha <= 0) {
particles.splice(index, 1);
}
});
requestAnimationFrame(render);
}
render();
```
这个代码会在画布上随机生成多个烟花,并且烟花会爆炸成多个颜色不同的粒子。你可以尝试修改代码中的参数,例如烟花的粒子数、颜色等,来实现不同的效果。
阅读全文
相关推荐
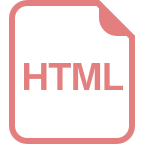
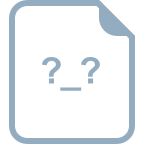
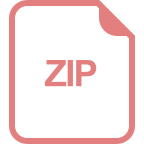
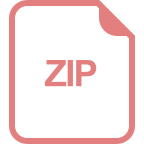
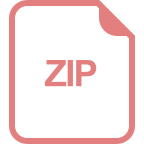
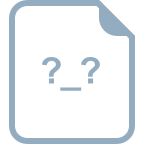
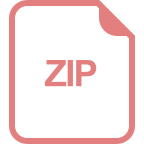
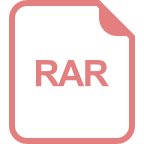
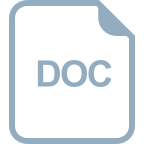
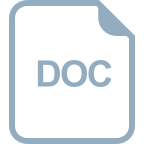
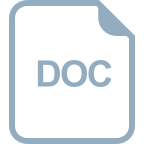
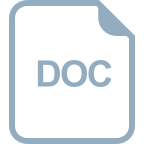
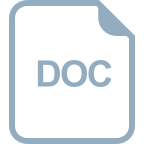


