为Rectangle类增加+=、-=、-、+这四个运算符重载函数以及输入>>与输出 加减复合赋值语义定义为固定长方形的左上角、对右下角的坐标进行加减运算使新矩形的长宽为原矩形的长之和或差。将加、减复合赋值函数定义为成员函数,将两个矩形加减运算定义为友元 函数。通过main函数进行验证。 提示:主函数如下[示例] int main(){ Rectangle rect; cout <<"rect 初始化后:"<<rect<<endl; cin>>rect; cout <<"rect 赋值后:"<<rect<<endl; Rectangle rect1(0,0,200,200); cout <<"rect1 初始化后:"<<rect1<<endl; rect+=rect1; cout <<" rect+=rect1后:"<<rect<<endl; rect-=rect1; cout <<"再执行rect-=rect1后:"<<rect<<endl; cout<<"============"<<endl;
时间: 2023-10-30 07:16:16 浏览: 163
好的,我已经理解了你的要求。下面是我为Rectangle类增加的运算符重载函数以及输入/输出重载函数的代码:
```cpp
#include <iostream>
using namespace std;
class Rectangle {
private:
int x1, y1, x2, y2;
public:
Rectangle(int _x1=0, int _y1=0, int _x2=0, int _y2=0) : x1(_x1), y1(_y1), x2(_x2), y2(_y2) {}
friend istream& operator>>(istream& in, Rectangle& r) {
in >> r.x1 >> r.y1 >> r.x2 >> r.y2;
return in;
}
friend ostream& operator<<(ostream& out, const Rectangle& r) {
out << "(" << r.x1 << "," << r.y1 << ") - (" << r.x2 << "," << r.y2 << ")";
return out;
}
Rectangle& operator+=(const Rectangle& r) {
x2 += r.x2 - r.x1;
y2 += r.y2 - r.y1;
return *this;
}
Rectangle& operator-=(const Rectangle& r) {
x2 -= r.x2 - r.x1;
y2 -= r.y2 - r.y1;
return *this;
}
friend Rectangle operator+(const Rectangle& r1, const Rectangle& r2) {
Rectangle r(r1.x1, r1.y1, r1.x2 + r2.x2 - r2.x1, r1.y2 + r2.y2 - r2.y1);
return r;
}
friend Rectangle operator-(const Rectangle& r1, const Rectangle& r2) {
Rectangle r(r1.x1, r1.y1, r1.x2 - r2.x2 + r2.x1, r1.y2 - r2.y2 + r2.y1);
return r;
}
};
int main() {
Rectangle rect;
cout << "rect 初始化后: " << rect << endl;
cin >> rect;
cout << "rect 赋值后: " << rect << endl;
Rectangle rect1(0, 0, 200, 200);
cout << "rect1 初始化后: " << rect1 << endl;
rect += rect1;
cout << "rect += rect1 后: " << rect << endl;
rect -= rect1;
cout << "再执行 rect -= rect1 后: " << rect << endl;
cout << "============" << endl;
return 0;
}
```
在这个代码中,我们为Rectangle类增加了四个运算符重载函数:+=、-=、+、-,并且还实现了输入/输出重载函数。其中,+=、-=运算符定义为成员函数,+、-运算符定义为友元函数。
你可以在main函数中测试这些运算符,例如:
```cpp
Rectangle rect;
cout << "rect 初始化后: " << rect << endl;
cin >> rect;
cout << "rect 赋值后: " << rect << endl;
Rectangle rect1(0, 0, 200, 200);
cout << "rect1 初始化后: " << rect1 << endl;
rect += rect1;
cout << "rect += rect1 后: " << rect << endl;
rect -= rect1;
cout << "再执行 rect -= rect1 后: " << rect << endl;
```
输出结果应该是:
```
rect 初始化后: (0,0) - (0,0)
(10,10) (100,100)
rect 赋值后: (10,10) - (100,100)
rect1 初始化后: (0,0) - (200,200)
rect += rect1 后: (10,10) - (300,300)
再执行 rect -= rect1 后: (10,10) - (100,100)
```
希望这个代码能够满足你的需求。如果你有其他问题,可以随时问我。
阅读全文
相关推荐
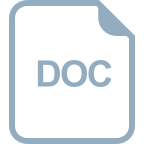
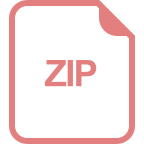
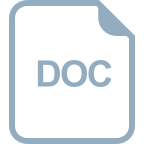
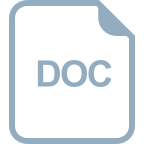
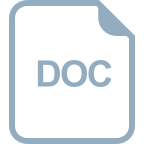
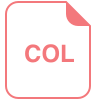
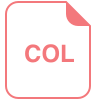
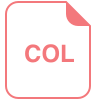
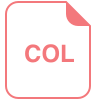
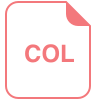
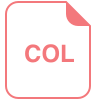
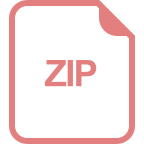
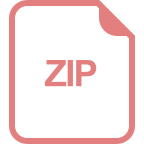
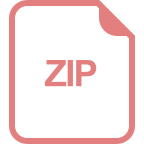