设链表结点结构已经给出,试编写链表插入函数Insert将实现链表末尾添加数据,再编写函数Print,实现链表中链表数据打印,编写Destroy函数,实现链表的销毁释放,并在main函数中写出完整的调用过程。
时间: 2023-07-11 12:41:41 浏览: 84
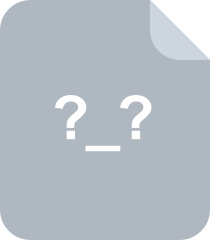
链表的建立,打印,删除结点
好的,我来为你编写这些函数。
首先,我们需要定义链表节点的结构体:
```c
typedef struct Node {
int data;
struct Node *next;
} Node;
```
然后,我们可以编写一个函数来插入节点。这里我们采用在链表末尾添加节点的方式:
```c
void Insert(Node **head, int data) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
Node *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = newNode;
}
}
```
接下来,我们需要编写一个函数来打印链表中的数据:
```c
void Print(Node *head) {
Node *p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
最后,我们需要编写一个函数来销毁链表。我们可以遍历整个链表,依次释放每个节点的内存空间:
```c
void Destroy(Node **head) {
Node *p = *head;
while (p != NULL) {
Node *q = p->next;
free(p);
p = q;
}
*head = NULL;
}
```
在main函数中,我们可以这样调用上面的函数:
```c
int main() {
Node *head = NULL;
// 插入节点
Insert(&head, 1);
Insert(&head, 2);
Insert(&head, 3);
// 打印链表中的数据
Print(head);
// 销毁链表
Destroy(&head);
return 0;
}
```
这样,我们就完成了链表插入、打印、销毁的操作。
阅读全文
相关推荐
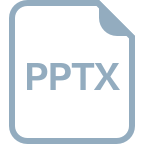
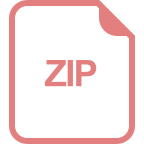
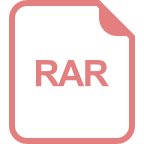
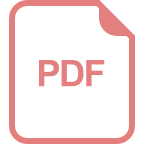
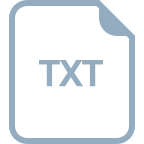
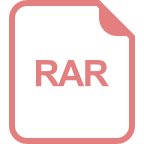
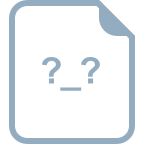
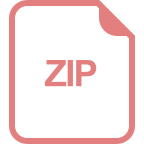
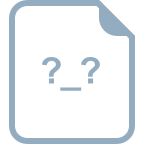
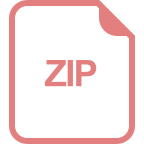
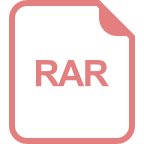