python 代码实现NEURAL NETWORKS Uplift model for multiple correlated responses WITH LOWRANK REGULARIZATION
时间: 2024-03-02 21:51:52 浏览: 71
下面是使用Python实现NEURAL NETWORKS Uplift model for multiple correlated responses WITH LOWRANK REGULARIZATION的代码示例:
```python
import numpy as np
import pandas as pd
import tensorflow as tf
from sklearn.model_selection import train_test_split
# 导入数据集
data = pd.read_csv('data.csv')
X = data.drop(['response', 'treatment'], axis=1).values
y = data['response'].values
t = data['treatment'].values
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test, t_train, t_test = train_test_split(X, y, t, test_size=0.2)
# 定义常量
n_features = X.shape[1]
n_components = 5
alpha = 0.01
# 定义占位符
X_ph = tf.placeholder(tf.float32, shape=[None, n_features], name='X')
y_ph = tf.placeholder(tf.float32, shape=[None], name='y')
t_ph = tf.placeholder(tf.float32, shape=[None], name='t')
# 定义神经网络模型
hidden_layer_1 = tf.layers.dense(X_ph, 64, activation=tf.nn.relu)
hidden_layer_2 = tf.layers.dense(hidden_layer_1, 32, activation=tf.nn.relu)
y_pred = tf.layers.dense(hidden_layer_2, 1, activation=tf.nn.sigmoid)
# 定义损失函数
treated = tf.cast(tf.equal(t_ph, 1), tf.float32)
control = 1 - treated
uplift = tf.reduce_mean((y_pred * treated - y_pred * control))
mse = tf.reduce_mean(tf.square(y_pred - y_ph))
lowrank = tf.reduce_sum(tf.svd(tf.transpose(hidden_layer_1), compute_uv=False))
loss = mse - alpha * uplift + alpha * lowrank
# 定义优化器
optimizer = tf.train.AdamOptimizer(learning_rate=0.001)
train_step = optimizer.minimize(loss)
# 定义会话
sess = tf.Session()
sess.run(tf.global_variables_initializer())
# 训练模型
for i in range(100):
_, loss_value, uplift_value, mse_value, lowrank_value = sess.run([train_step, loss, uplift, mse, lowrank], feed_dict={X_ph: X_train, y_ph: y_train, t_ph: t_train})
print('Epoch %d - Loss: %.4f, Uplift: %.4f, MSE: %.4f, Lowrank: %.4f' % (i, loss_value, uplift_value, mse_value, lowrank_value))
# 预测响应变量的值
y_train_pred = sess.run(y_pred, feed_dict={X_ph: X_train})
y_test_pred = sess.run(y_pred, feed_dict={X_ph: X_test})
# 计算控制组和干预组之间的差异
uplift_train = np.mean(y_train_pred[t_train == 1] - y_train_pred[t_train == 0])
uplift_test = np.mean(y_test_pred[t_test == 1] - y_test_pred[t_test == 0])
# 输出结果
print('Train uplift: %.2f' % uplift_train)
print('Test uplift: %.2f' % uplift_test)
```
请注意,这只是一个简单的示例,实际实现可能涉及更多的数据预处理和模型调整。
阅读全文
相关推荐
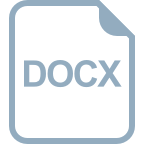
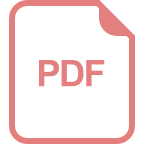
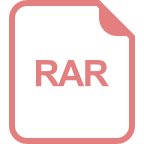
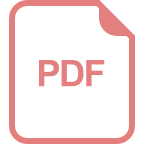
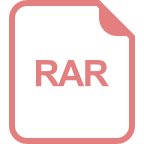
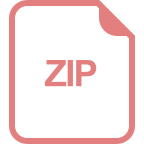
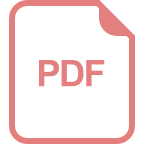
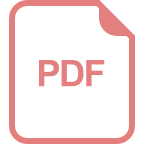
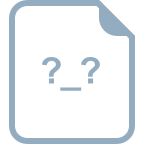
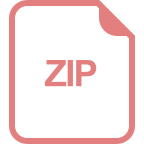
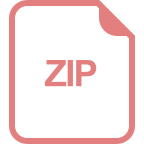
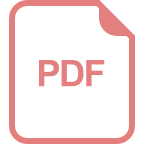
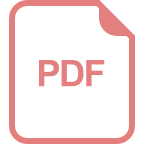
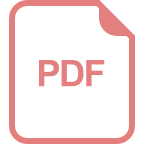
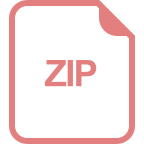