给我代码:django读取后端文件夹中的图片并返回URL给前端,且图片显示在前端页面
时间: 2024-03-23 22:42:30 浏览: 87
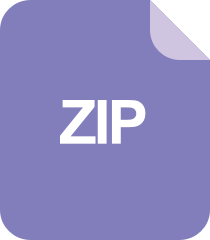
基于Django+MySQL+python实现的简易的天气预报系统源码(毕业设计项目).zip

好的,以下是一个简单的示例代码,展示如何使用 Django 从后端文件夹中读取图片并返回 URL 给前端,同时在前端页面中显示图片:
1. 在 Django 项目中创建一个 app,例如 `myapp`
2. 在 `views.py` 文件中编写视图函数,从文件夹中读取图片并返回 URL 给前端。例如:
```python
from django.conf import settings
from django.http import JsonResponse
import os
def image_list(request):
image_dir = os.path.join(settings.BASE_DIR, 'myapp/images')
image_urls = []
for filename in os.listdir(image_dir):
if filename.endswith('.jpg') or filename.endswith('.png'):
image_urls.append(request.build_absolute_uri('/images/' + filename))
return JsonResponse({'image_urls': image_urls})
```
3. 在 `urls.py` 文件中配置路由,将视图函数映射到 URL。例如:
```python
from django.urls import path
from . import views
urlpatterns = [
path('images/', views.image_list, name='image_list'),
]
```
4. 在 `settings.py` 文件中配置静态文件路径和 URL。例如:
```python
STATIC_URL = '/static/'
STATICFILES_DIRS = [os.path.join(BASE_DIR, 'static')]
MEDIA_URL = '/media/'
MEDIA_ROOT = os.path.join(BASE_DIR, 'media')
```
5. 在前端页面中使用 JavaScript 代码,从后端 API 中获取图片 URL 并显示图片。例如:
```html
<!DOCTYPE html>
<html>
<head>
<title>Image List</title>
</head>
<body>
<h1>Image List</h1>
<div id="image-container"></div>
<script>
fetch('/images/')
.then(response => response.json())
.then(data => {
const container = document.getElementById('image-container');
data.image_urls.forEach(url => {
const img = document.createElement('img');
img.src = url;
container.appendChild(img);
});
});
</script>
</body>
</html>
```
6. 运行 Django 项目,访问 `http://localhost:8000/images/` 可以看到返回的图片 URL 列表,同时在前端页面中可以看到图片。
希望这个示例代码可以帮到你!
阅读全文
相关推荐
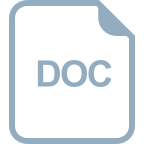
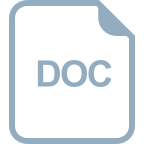
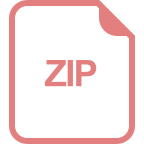
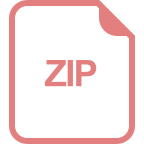
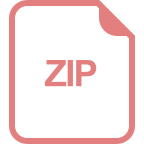
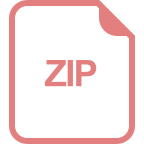
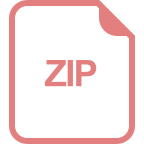
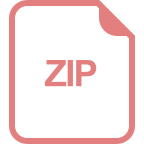
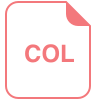
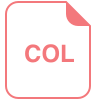
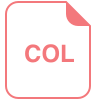
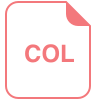
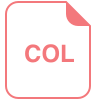
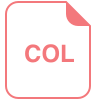
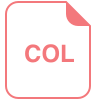
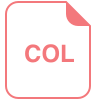
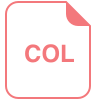
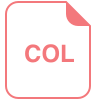