用c语言写个stl的list
时间: 2023-09-11 10:05:12 浏览: 81
### 回答1:
STL(Standard Template Library)是 C++ 语言的标准模板库,而不是 C 语言。因此,如果您想在 C 语言中使用 STL 的 list,您可以写一个类似的数据结构,例如链表。
下面是一个简单的链表实现:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
Node *create_node(int data) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = data;
new_node->next = NULL;
return new_node;
}
void insert_node(Node **head, int data) {
Node *new_node = create_node(data);
new_node->next = *head;
*head = new_node;
}
void print_list(Node *head) {
Node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
Node *head = NULL;
insert_node(&head, 1);
insert_node(&head, 2);
insert_node(&head, 3);
print_list(head);
return 0;
}
```
输出结果为:
```
3 2 1
```
请注意,这只是一个简单的链表实现,并不是 STL 的 list 的完整实现,但它可以作为您开始学习链表的一个起点。
### 回答2:
在C语言中实现一个类似STL的list可以通过使用指针和结构体来完成。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
struct Node* prev;
} Node;
typedef struct {
Node* head;
Node* tail;
} List;
List* createList() {
List* list = (List*)malloc(sizeof(List));
list->head = NULL;
list->tail = NULL;
return list;
}
void insert(List* list, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
newNode->prev = NULL;
if (list->head == NULL) {
// Inserting into an empty list
list->head = newNode;
list->tail = newNode;
} else {
// Inserting at the end of the list
list->tail->next = newNode;
newNode->prev = list->tail;
list->tail = newNode;
}
}
void display(List* list) {
Node* current = list->head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
List* list = createList();
insert(list, 10);
insert(list, 20);
insert(list, 30);
display(list);
return 0;
}
```
上述代码使用了两个结构体,Node表示每个节点,List表示整个链表。函数createList()用于创建一个新的链表,insert()函数用于将元素插入到链表的末尾,display()函数用于显示链表中的所有元素。
在main()函数中,我们创建了一个新的链表,然后插入了三个元素,最后调用display()函数显示链表中的所有元素。输出结果为:10 20 30。这个简单的C语言示例实现了最基本的链表操作,你可以根据需要进一步扩展功能,实现更完整的STL list。
### 回答3:
C语言是一种非面向对象的编程语言,因此它不直接支持STL(标准模板库)的容器,比如list。不过我们可以通过使用指针和动态内存分配来实现类似于list的数据结构。
要实现一个类似于STL的list,我们可以创建一个结构体来表示list的节点,其中包含一个数据成员和两个指针成员,分别指向前一个节点和后一个节点。同时,我们还需要定义一些用于操作list的函数。
首先,我们需要定义一个创建list节点的函数,它接受一个数据作为参数,并返回指向创建节点的指针。代码如下所示:
```
typedef struct ListNode {
int data;
struct ListNode* prev;
struct ListNode* next;
} ListNode;
ListNode* createNode(int data) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = data;
newNode->prev = NULL;
newNode->next = NULL;
return newNode;
}
```
接下来,我们可以定义一个结构体来表示list本身,其中包含指向第一个节点和最后一个节点的指针。我们还可以定义一些用于操作list的函数,比如插入节点、删除节点、获取list长度等。这些函数可以根据具体需求进行实现。
以下是一个简单的示例代码,演示了如何使用C语言来实现类似于STL的list:
```
typedef struct List {
ListNode* head;
ListNode* tail;
} List;
void insertNode(List* list, int data) {
ListNode* newNode = createNode(data);
if (list->head == NULL) {
list->head = newNode;
list->tail = newNode;
} else {
newNode->prev = list->tail;
list->tail->next = newNode;
list->tail = newNode;
}
}
void deleteNode(List* list, int data) {
ListNode* currentNode = list->head;
while (currentNode != NULL) {
if (currentNode->data == data) {
if (currentNode->prev != NULL) {
currentNode->prev->next = currentNode->next;
} else {
list->head = currentNode->next;
}
if (currentNode->next != NULL) {
currentNode->next->prev = currentNode->prev;
} else {
list->tail = currentNode->prev;
}
free(currentNode);
break;
}
currentNode = currentNode->next;
}
}
int getListLength(List* list) {
int length = 0;
ListNode* currentNode = list->head;
while (currentNode != NULL) {
length++;
currentNode = currentNode->next;
}
return length;
}
```
通过以上代码,我们可以实现一个简单的类似于STL的list,它包含插入节点、删除节点和获取list长度等操作。当然,这只是一个简单的实现示例,实际使用时还可能需要考虑更多的细节和功能。
相关推荐
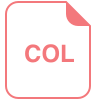
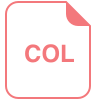
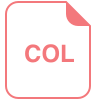











