tcp拥塞控制算法代码
时间: 2023-07-09 14:29:29 浏览: 161
以下是TCP Reno算法的简单实现代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_PACKETS 1000 // 最大数据包数量
#define MSS 512 // 最大段大小
#define RTT 200 // 往返时间
#define ALPHA 0.125 // 慢启动下降因子
#define BETA 0.25 // 拥塞避免下降因子
#define THRESHOLD 16 // 拥塞阈值
// TCP Reno算法状态
typedef enum {
SLOW_START,
CONGESTION_AVOIDANCE,
FAST_RECOVERY
} TCP_Reno_State;
// 数据包结构体
typedef struct {
int seq_num; // 序列号
int size; // 数据包大小
} Packet;
// 计算超时时间
int timeout() {
return 2 * RTT;
}
// 慢启动算法
int slow_start(int cwnd, int ssthresh) {
cwnd += MSS;
if (cwnd >= ssthresh) {
return CONGESTION_AVOIDANCE;
}
return SLOW_START;
}
// 拥塞避免算法
int congestion_avoidance(int cwnd, int ssthresh) {
cwnd += MSS * (MSS / cwnd);
if (cwnd >= ssthresh) {
return CONGESTION_AVOIDANCE;
}
return SLOW_START;
}
// 快速恢复算法
int fast_recovery(int cwnd, int ssthresh) {
cwnd = ssthresh;
return CONGESTION_AVOIDANCE;
}
// TCP Reno算法
int tcp_reno(Packet packets[], int num_packets) {
int cwnd = MSS;
int ssthresh = THRESHOLD * MSS;
int state = SLOW_START;
int acked = 0;
int dup_ack_count = 0;
int i;
for (i = 0; i < num_packets; i++) {
// 接收到确认号
if (packets[i].seq_num <= acked) {
continue;
}
acked = packets[i].seq_num;
// 慢启动状态下
if (state == SLOW_START) {
state = slow_start(cwnd, ssthresh);
}
// 拥塞避免状态下
else if (state == CONGESTION_AVOIDANCE) {
state = congestion_avoidance(cwnd, ssthresh);
}
// 快速恢复状态下
else if (state == FAST_RECOVERY) {
cwnd += MSS;
state = CONGESTION_AVOIDANCE;
}
// 重传数据包
if (dup_ack_count >= 3) {
ssthresh = cwnd / 2;
cwnd = ssthresh + 3 * MSS;
state = FAST_RECOVERY;
dup_ack_count = 0;
continue;
}
// 发送数据包
while (cwnd >= packets[i].size) {
cwnd -= packets[i].size;
}
// 接收到重复确认号
if (packets[i].seq_num == acked) {
dup_ack_count++;
continue;
}
// 更新拥塞窗口大小
if (state == SLOW_START) {
cwnd += MSS;
} else if (state == CONGESTION_AVOIDANCE) {
cwnd += MSS * (MSS / cwnd);
}
}
return 0;
}
// 主函数
int main() {
Packet packets[MAX_PACKETS];
int num_packets;
int i;
for (i = 0; i < num_packets; i++) {
packets[i].seq_num = i;
packets[i].size = MSS;
}
tcp_reno(packets, num_packets);
return 0;
}
```
阅读全文
相关推荐
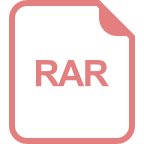















