vue 数组根据下标分组
时间: 2023-11-17 14:06:45 浏览: 29
可以使用 JavaScript 的 reduce 方法来实现根据下标分组:
```
const arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const result = arr.reduce((acc, val, idx) => {
const groupIndex = Math.floor(idx / 3);
acc[groupIndex] = acc[groupIndex] || [];
acc[groupIndex].push(val);
return acc;
}, []);
console.log(result); // [[1, 2, 3], [4, 5, 6], [7, 8, 9], [10]]
```
这里的 reduce 方法的第二个参数是一个空数组,作为初始值。在 reduce 的回调函数中,将数组元素按照下标分组,每 3 个元素为一组。将分组后的结果存储在累加器(acc)中,并在每次迭代时返回 acc。最终得到的结果是一个包含 4 个子数组的数组。
相关问题
vue 获取数组的下标
要获取一个元素在数组中的下标,可以使用 JavaScript 中的 indexOf() 方法或 findIndex() 方法。
indexOf() 方法用于查找数组中给定元素的第一个匹配项,并返回其在数组中的索引。例如:
```
const items = ['apple', 'banana', 'orange'];
const index = items.indexOf('banana'); // 获取 'banana' 在数组中的索引,即 1
```
findIndex() 方法用于查找数组中满足条件的第一个元素,并返回其在数组中的索引。例如:
```
const items = [
{ name: 'apple', price: 1.5 },
{ name: 'banana', price: 2.0 },
{ name: 'orange', price: 1.0 },
];
const index = items.findIndex(item => item.name === 'banana'); // 获取 name 为 'banana' 的元素在数组中的索引,即 1
```
在这个例子中,我们定义了一个包含多个对象的数组 items,每个对象都有一个 name 属性。我们要查找 name 为 'banana' 的元素在数组中的索引,所以我们使用 findIndex() 方法来查找数组中第一个 name 属性等于 'banana' 的元素,并将其索引存储在 index 变量中。
vue数组下标改变页面没有实时刷新
在 Vue 中,当你修改数组的下标时,Vue 并不会检测到数组的变化。这是因为 Vue 的响应式系统通过劫持数组的方法来实现的,当你修改数组的下标时,它无法检测到这个变化。
解决这个问题有两种方法:
1. 使用 Vue.set 或者 this.$set 方法来修改数组。这个方法可以让 Vue 监听到数组的变化,从而实现实时更新。例如:
```
this.$set(myArray, index, newValue);
```
2. 使用 Vue.set 或者 this.$set 方法来添加或者删除数组元素。这个方法可以让 Vue 监听到数组的变化,从而实现实时更新。例如:
```
this.$set(myArray, index, newValue);
this.$splice(myArray, index, 1);
```
以上两种方法都可以让 Vue 监听到数组的变化,并实现实时更新。但是需要注意的是,这种方法可能会导致性能问题,因为它会频繁地触发 Vue 的重新渲染。如果你的数组非常大,这种方法可能会导致页面卡顿。因此,你应该尽可能地避免修改数组下标,或者使用更高效的数据结构来管理你的数据。
相关推荐
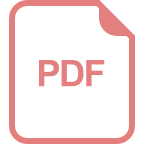












