Pytorch搭建一个房价预测模型
时间: 2024-05-14 08:14:48 浏览: 13
以下是使用Pytorch搭建一个房价预测模型的步骤:
1. 导入必要的库
```python
import torch
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
```
2. 加载数据集
```python
data = pd.read_csv('housing.csv')
```
3. 数据预处理
```python
# 将数据分为特征和目标
features = data.iloc[:, :-1]
target = data.iloc[:, -1]
# 数据标准化
features = (features - features.mean()) / features.std()
# 将特征和目标转换为NumPy数组
features = np.array(features)
target = np.array(target)
target = target.reshape(-1, 1)
```
4. 将数据集分为训练集和测试集
```python
# 将数据集分为训练集和测试集,比例为80:20
from sklearn.model_selection import train_test_split
train_features, test_features, train_target, test_target = train_test_split(features, target, test_size=0.2, random_state=42)
```
5. 定义模型
```python
class LinearRegression(torch.nn.Module):
def __init__(self, input_size, output_size):
super(LinearRegression, self).__init__()
self.linear = torch.nn.Linear(input_size, output_size)
def forward(self, x):
return self.linear(x)
```
6. 实例化模型
```python
model = LinearRegression(train_features.shape[1], 1)
```
7. 定义损失函数和优化器
```python
criterion = torch.nn.MSELoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
```
8. 训练模型
```python
num_epochs = 1000
for epoch in range(num_epochs):
# 将训练集转换为张量
inputs = torch.from_numpy(train_features).float()
targets = torch.from_numpy(train_target).float()
# 清零梯度
optimizer.zero_grad()
# 前向传播
outputs = model(inputs)
# 计算损失
loss = criterion(outputs, targets)
# 反向传播和优化
loss.backward()
optimizer.step()
# 每100个epoch输出一次损失
if (epoch+1) % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
```
9. 测试模型
```python
# 将测试集转换为张量
inputs = torch.from_numpy(test_features).float()
# 预测房价
predicted = model(inputs).data.numpy()
# 计算均方根误差
from sklearn.metrics import mean_squared_error
mse = mean_squared_error(test_target, predicted)
rmse = np.sqrt(mse)
print('RMSE: {:.4f}'.format(rmse))
```
10. 可视化结果
```python
plt.plot(test_target, label='Actual')
plt.plot(predicted, label='Predicted')
plt.legend()
plt.show()
```
完成以上步骤后,就可以使用Pytorch搭建一个房价预测模型了。
相关推荐
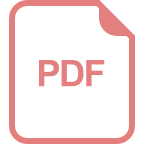











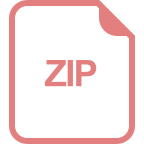
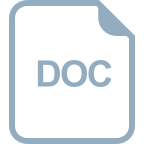
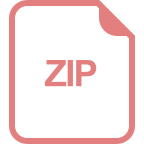
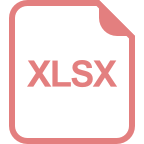