c++利用YUV图像计算坏点,怎么实现
时间: 2024-01-07 15:03:09 浏览: 88
计算坏点需要对YUV图像进行处理。具体实现步骤如下:
1. 读取YUV图像,将数据存储在一个数组中。
2. 遍历数组中的每一个像素点,计算该像素点的亮度值Y。
3. 判断该像素点是否为坏点。一般情况下,坏点的亮度值会偏离正常值,所以可以根据一定的阈值来判断该像素点是否为坏点。如果该像素点的亮度值小于阈值,则认为该像素点为坏点。
4. 对于坏点,需要进行修复。一般的修复方法是使用周围像素点的平均值来取代坏点。具体地,可以找到坏点周围一定范围内的正常像素点,然后计算这些像素点的平均值,将平均值作为坏点的修复值。
5. 将修复后的YUV图像保存到文件中。
下面是C++代码实现:
```
#include <iostream>
#include <fstream>
using namespace std;
const int WIDTH = 640; // 图像宽度
const int HEIGHT = 480; // 图像高度
const int THRESHOLD = 50; // 判断坏点的阈值
const int RADIUS = 3; // 坏点修复半径
// 读取YUV图像
void read_yuv(const char* file, unsigned char* yuv) {
ifstream in(file, ios::binary);
in.read(reinterpret_cast<char*>(yuv), WIDTH * HEIGHT * 3 / 2);
in.close();
}
// 写入YUV图像
void write_yuv(const char* file, const unsigned char* yuv) {
ofstream out(file, ios::binary);
out.write(reinterpret_cast<const char*>(yuv), WIDTH * HEIGHT * 3 / 2);
out.close();
}
// 计算像素点是否为坏点
bool is_bad_pixel(unsigned char* yuv, int x, int y) {
int pos = y * WIDTH + x;
int y_val = yuv[pos];
return (y_val < THRESHOLD);
}
// 修复坏点
void fix_bad_pixel(unsigned char* yuv, int x, int y) {
int sum = 0;
int count = 0;
// 计算周围像素点的平均值
for (int i = x - RADIUS; i <= x + RADIUS; i++) {
for (int j = y - RADIUS; j <= y + RADIUS; j++) {
if (i >= 0 && i < WIDTH && j >= 0 && j < HEIGHT && !is_bad_pixel(yuv, i, j)) {
int pos = j * WIDTH + i;
sum += yuv[pos];
count++;
}
}
}
if (count > 0) {
int pos = y * WIDTH + x;
yuv[pos] = sum / count; // 用平均值修复坏点
}
}
// 处理YUV图像
void process_yuv(unsigned char* yuv) {
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
if (is_bad_pixel(yuv, x, y)) {
fix_bad_pixel(yuv, x, y);
}
}
}
}
int main() {
unsigned char* yuv = new unsigned char[WIDTH * HEIGHT * 3 / 2];
read_yuv("input.yuv", yuv); // 读取YUV图像
process_yuv(yuv); // 处理YUV图像
write_yuv("output.yuv", yuv); // 写入YUV图像
delete[] yuv;
return 0;
}
```
阅读全文
相关推荐
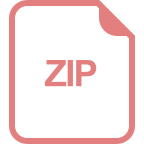
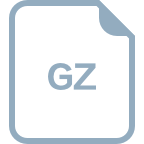
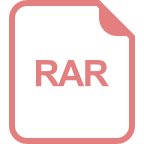
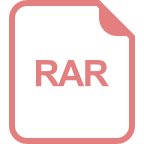
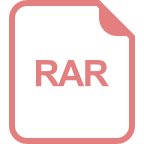
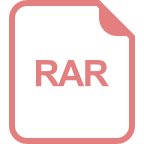
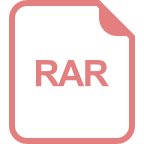
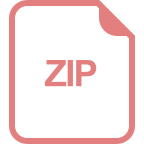
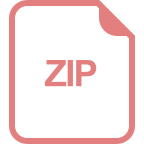
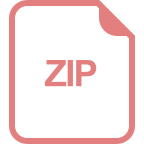
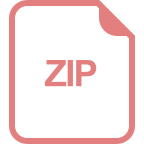
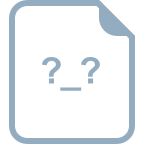
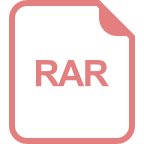
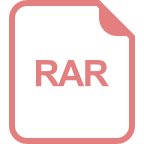
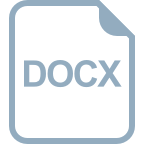
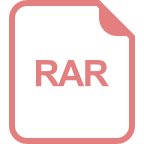
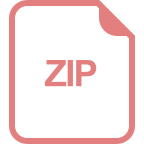
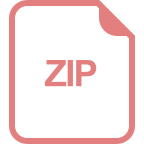