#include <iostream> using namespace std; int main() { int a{}; for (int a = 1; a < 100; a++); { if (a % 10 == 7 / (a - a % 10) % 7 == 0 / a % 7 == 0) cout << a << endl; } system("pause"); return 0; }
时间: 2024-01-14 10:03:05 浏览: 74
这段代码存在一些问题,其中最明显的是语法错误。以下是修正后的代码:
```c++
#include <iostream>
using namespace std;
int main() {
for (int a = 1; a < 100; a++) {
if (a % 10 == 7 && (a - a % 10) % 7 == 0 && a % 7 == 0) {
cout << a << endl;
}
}
system("pause");
return 0;
}
```
修正后的代码使用了大括号将 `for` 循环体括起来,并且在 `if` 语句中使用了逻辑运算符 `&&` 来连接多个条件。注意,这里的 `7 / (a - a % 10)` 应该改为 `(a - a % 10) % 7 == 0`,以确保语法正确。
相关问题
#include<iostream> #include<random> using namespace std; int main(){ int a = random(1,100); cout<<a; }
这个C++代码片段尝试使用`std`命名空间,并从头文件`#include <iostream>`和`#include <random>`导入必要的库。但是,实际上在C++11标准及以后版本中,`random()`函数已经不再直接存在于`std`命名空间内,而是应该使用`std::mt19937`等随机数引擎配合`std::uniform_int_distribution`来生成随机数。
正确的写法应该是:
```cpp
#include <iostream>
#include <random>
using namespace std;
int main() {
// 使用Mersenne Twister算法作为随机数引擎
mt19937 engine; // 需要先实例化一个随机数引擎
// 设定随机数的范围
uniform_int_distribution<int> dist(1, 100); // 注意是int而不是random
// 生成随机数并输出
int a = dist(engine);
cout << "Random number between 1 and 100: " << a << endl;
return 0;
}
```
在上述代码中,`dist(engine)`会生成1到100之间的随机整数。
#include <iostream> using namespace std; int main()
你提供的代码片段是一个基本的C++程序的开始部分。它包含了iostream头文件,并使用了命名空间std。main函数是程序的入口点,它是程序执行的起始位置。
然而,你的代码片段是不完整的,缺少了main函数的实现部分。在main函数中,你可以编写程序的逻辑代码,执行各种操作和输出。
以下是一个完整的示例程序,展示了如何使用iostream和main函数:
```c++
#include <iostream>
using namespace std;
int main()
{
// 在这里编写你的程序逻辑代码
cout << "Hello, World!" << endl; // 示例输出语句
return 0;
}
```
在这个示例中,我们在main函数中输出了一条简单的问候语句"Hello, World!",然后返回0表示程序正常结束。
你可以在main函数中编写任意的C++代码来实现你的需求。如果你有特定的问题或需要进一步的帮助,请告诉我具体需要做什么,我会尽力提供帮助。
阅读全文
相关推荐
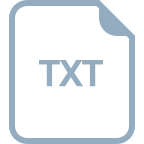
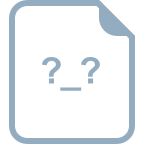
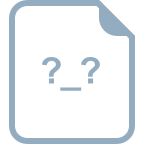













