C++窗体实现成语字典查询
时间: 2023-07-13 16:26:22 浏览: 67
您好,以下是一个简单的 C++ 窗体程序实现成语字典查询的示例代码。
首先,需要使用 Visual Studio 创建一个 Win32 窗体应用程序项目。
在 Form1.h 文件中,添加如下代码:
```c++
#pragma once
#include <string>
#include <vector>
#include <algorithm>
#include <fstream>
namespace IdiomDictionary {
struct Idiom {
std::string name;
std::string explanation;
};
class Form1 : public System::Windows::Forms::Form {
private:
std::vector<Idiom> m_idioms;
public:
Form1() {
InitializeComponent();
}
protected:
~Form1() {
if (components) {
delete components;
}
}
private:
System::Windows::Forms::TextBox^ textBox1;
System::Windows::Forms::Button^ button1;
System::Windows::Forms::ListBox^ listBox1;
System::ComponentModel::Container^ components;
void InitializeComponent(void) {
this->textBox1 = (gcnew System::Windows::Forms::TextBox());
this->button1 = (gcnew System::Windows::Forms::Button());
this->listBox1 = (gcnew System::Windows::Forms::ListBox());
this->SuspendLayout();
//
// textBox1
//
this->textBox1->Location = System::Drawing::Point(12, 12);
this->textBox1->Name = L"textBox1";
this->textBox1->Size = System::Drawing::Size(200, 21);
this->textBox1->TabIndex = 0;
//
// button1
//
this->button1->Location = System::Drawing::Point(218, 12);
this->button1->Name = L"button1";
this->button1->Size = System::Drawing::Size(75, 23);
this->button1->TabIndex = 1;
this->button1->Text = L"查询";
this->button1->UseVisualStyleBackColor = true;
this->button1->Click += gcnew System::EventHandler(this, &Form1::button1_Click);
//
// listBox1
//
this->listBox1->FormattingEnabled = true;
this->listBox1->Location = System::Drawing::Point(12, 39);
this->listBox1->Name = L"listBox1";
this->listBox1->Size = System::Drawing::Size(281, 212);
this->listBox1->TabIndex = 2;
//
// Form1
//
this->AutoScaleDimensions = System::Drawing::SizeF(6, 12);
this->AutoScaleMode = System::Windows::Forms::AutoScaleMode::Font;
this->ClientSize = System::Drawing::Size(308, 261);
this->Controls->Add(this->listBox1);
this->Controls->Add(this->button1);
this->Controls->Add(this->textBox1);
this->Name = L"Form1";
this->Text = L"成语字典查询";
this->Load += gcnew System::EventHandler(this, &Form1::Form1_Load);
this->ResumeLayout(false);
this->PerformLayout();
}
void button1_Click(System::Object^ sender, System::EventArgs^ e) {
std::string keyword = msclr::interop::marshal_as<std::string>(textBox1->Text);
std::vector<std::string> results;
for (auto& idiom : m_idioms) {
if (idiom.name.find(keyword) != std::string::npos) {
results.push_back(idiom.name + ":" + idiom.explanation);
}
}
listBox1->Items->Clear();
listBox1->Items->AddRange(gcnew cli::array<System::Object^>(results.size()));
for (int i = 0; i < results.size(); ++i) {
listBox1->Items[i] = gcnew System::String(results[i].c_str());
}
}
void Form1_Load(System::Object^ sender, System::EventArgs^ e) {
std::ifstream file("idiom.txt");
std::string line;
while (std::getline(file, line)) {
size_t pos = line.find(":");
if (pos != std::string::npos) {
Idiom idiom;
idiom.name = line.substr(0, pos);
idiom.explanation = line.substr(pos + 1);
m_idioms.push_back(idiom);
}
}
file.close();
}
};
}
```
其中,我们使用了一个 Idiom 结构体来存储每个成语的名称和解释。在 Form1 类中,我们使用了一个 std::vector 来存储所有的成语,并在窗体加载时从文本文件中读取。在查询时,我们遍历所有的成语,找到包含关键字的成语,并将结果显示在 ListBox 控件中。
在 main.cpp 文件中,添加如下代码:
```c++
#include "Form1.h"
using namespace System;
using namespace System::Windows::Forms;
[STAThread]
int main(array<System::String^>^ args) {
Application::EnableVisualStyles();
Application::SetCompatibleTextRenderingDefault(false);
IdiomDictionary::Form1 form;
Application::Run(% form);
return 0;
}
```
这里我们简单地创建一个 Form1 对象,并将其传递给 Application::Run 方法来启动窗体应用程序。
最后,在项目中添加一个名为 idiom.txt 的文本文件,将所有的成语和解释按照“成语名:解释”的格式保存在文件中。
编译并运行程序,即可开始查询成语。
相关推荐
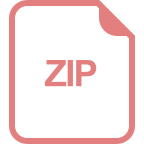
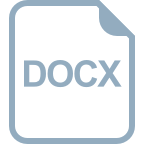
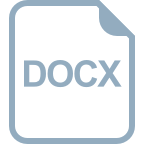














