c语言程序设计软件win10
时间: 2023-09-30 18:05:13 浏览: 49
有很多种C语言程序设计软件可以在Win10上使用,以下是其中一些常用的:
1. Visual Studio:由微软公司开发的集成开发环境(IDE),支持多种编程语言,包括C语言。
2. Code::Blocks:一个开源的跨平台C/C++集成开发环境,支持多种编译器和操作系统。
3. Dev-C++:一个免费的集成开发环境,支持C/C++编程,可在Windows操作系统上运行。
4. Eclipse:一个跨平台的集成开发环境,支持多种编程语言,包括C语言。
5. CodeLite:一个免费的开源跨平台C/C++集成开发环境,支持多种编译器和操作系统。
6. Turbo C++:一个老旧的C++编译器,适用于DOS和Windows操作系统。
以上是一些常用的C语言程序设计软件,选择合适的软件可以提高编程效率和质量。
相关问题
c语言程序设计21点扑克牌,C语言程序设计-21点扑克牌游戏.pdf
很抱歉,我无法提供您所需的pdf文件。但是,我可以为您提供一个简单的21点扑克牌游戏的C语言程序示例,您可以参考它来编写自己的程序。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int player, dealer, player_sum, dealer_sum;
char choice;
srand((unsigned)time(NULL)); // 初始化随机数种子
printf("Welcome to 21-point blackjack game!\n\n");
// 玩家和庄家各发两张牌
player = rand() % 10 + 1;
dealer = rand() % 10 + 1;
printf("Your first card is %d.\n", player);
printf("Dealer's first card is %d.\n", dealer);
player += rand() % 10 + 1;
dealer += rand() % 10 + 1;
printf("Your second card is %d.\n", player);
printf("Dealer's second card is hidden.\n");
// 计算点数
player_sum = player;
dealer_sum = dealer;
// 玩家回合
do {
printf("\nDo you want to hit (h) or stand (s)? ");
scanf(" %c", &choice);
if (choice == 'h') {
player += rand() % 10 + 1;
player_sum += player;
printf("Your new card is %d.\n", player);
if (player_sum > 21) {
printf("Busted! You lose.\n");
return 0;
}
}
} while (choice == 'h');
// 庄家回合
printf("\nDealer's turn.\n");
printf("Dealer's second card is %d.\n", dealer);
while (dealer_sum < 17) {
dealer += rand() % 10 + 1;
dealer_sum += dealer;
printf("Dealer's new card is %d.\n", dealer);
if (dealer_sum > 21) {
printf("Dealer busted! You win.\n");
return 0;
}
}
// 比较点数
printf("\nYour total is %d.\n", player_sum);
printf("Dealer's total is %d.\n", dealer_sum);
if (player_sum > dealer_sum) {
printf("You win!\n");
} else if (player_sum < dealer_sum) {
printf("You lose.\n");
} else {
printf("It's a tie.\n");
}
return 0;
}
```
这个程序使用随机数生成玩家和庄家的牌,在玩家回合中,玩家可以选择是否要继续要牌,直到选择停牌或者点数超过21点为止。在庄家回合中,庄家会一直要牌直到点数大于等于17点为止。最后比较点数大小,判断胜负。
请注意,这只是一个简单的示例程序,您可以根据自己的需求进行修改和优化。
32位win_tc c语言教材
32位Win_Tc是一种C语言教材,适用于在Windows操作系统中学习和编写C语言程序的教材。它为学习者提供了一个基于Windows环境的开发平台,方便他们进行实践和调试。
32位Win_Tc的教材内容通常包括C语言的基础知识、语法规则、变量和数据类型、控制结构、函数和指针等等。通过学习这些内容,学习者可以逐步掌握C语言的核心概念和编程技巧。
与传统的文本教材相比,32位Win_Tc提供了一个图形化的界面,使得学习者可以直观地进行程序设计和调试。它通常配有一个集成开发环境(IDE),其中包含了编辑器、编译器和调试器等工具,学习者可以通过这些工具快速编写、调试和执行自己的C语言程序。
此外,32位Win_Tc还可以与其他系统和软件进行集成,提供了丰富的库函数和API接口,使得学习者可以利用Windows操作系统提供的各种功能和资源进行开发。例如,学习者可以使用32位Win_Tc来编写窗口程序、图形界面、网络应用等。
总的来说,32位Win_Tc作为一种C语言教材,为学习者提供了一个在Windows环境下学习和实践C语言编程的平台。通过学习32位Win_Tc,学习者可以从基础知识到实际应用逐步提升自己的编程能力,并为将来的软件开发做好准备。
相关推荐
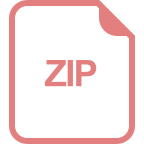













