实现linux下的基于C语言的evhttp请求中一个完整的POST的功能
时间: 2024-03-29 18:40:41 浏览: 13
要实现linux下的基于C语言的evhttp请求中一个完整的POST的功能,可以按照以下步骤进行:
1. 创建一个evhttp_connection对象,并设置连接的主机名和端口号。
2. 创建一个evhttp_request对象,并设置请求的方法为POST,请求的URI路径和查询参数。
3. 设置请求头部信息,包括Content-Type和Content-Length等。
4. 将POST请求的数据写入请求体中。
5. 发送POST请求并等待响应。
6. 处理响应结果,包括状态码、响应头部和响应体。
下面是一个示例代码,可以参考实现:
```c
#include <event2/event.h>
#include <event2/http.h>
#include <event2/buffer.h>
void http_request_done(struct evhttp_request *req, void *arg)
{
char *response = NULL;
int response_len = 0;
if (req == NULL) {
printf("http request failed.\n");
return;
}
if (req->response_code != HTTP_OK) {
printf("http response code: %d\n", req->response_code);
return;
}
struct evbuffer *evbuf = evhttp_request_get_input_buffer(req);
response_len = evbuffer_get_length(evbuf);
if (response_len > 0) {
response = (char*)malloc(response_len + 1);
evbuffer_remove(evbuf, response, response_len);
response[response_len] = 0;
printf("http response: %s\n", response);
}
}
int main(int argc, char **argv)
{
struct event_base *base = NULL;
struct evhttp_connection *conn = NULL;
struct evhttp_request *req = NULL;
const char *host = "www.example.com";
const int port = 80;
const char *path = "/api/test";
const char *data = "param1=value1¶m2=value2";
base = event_base_new();
conn = evhttp_connection_base_new(base, NULL, host, port);
req = evhttp_request_new(http_request_done, NULL);
evhttp_add_header(req->output_headers, "Content-Type", "application/x-www-form-urlencoded");
evhttp_add_header(req->output_headers, "Content-Length", strlen(data));
evbuffer_add(req->output_buffer, data, strlen(data));
evhttp_make_request(conn, req, EVHTTP_REQ_POST, path);
event_base_dispatch(base);
if (req != NULL) {
evhttp_request_free(req);
}
if (conn != NULL) {
evhttp_connection_free(conn);
}
if (base != NULL) {
event_base_free(base);
}
return 0;
}
```
这段代码演示了如何向www.example.com的/api/test路径发送一个POST请求,并传递参数param1=value1和param2=value2。在请求头部中设置了Content-Type和Content-Length信息,然后将请求数据写入请求体中。最后,通过调用event_base_dispatch函数等待响应结果,并在回调函数http_request_done中处理响应结果。
相关推荐
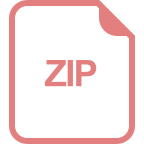
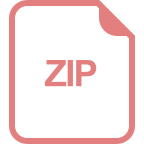














