java数字序列是一系列值,其中每个元素的值取决于一个或多个先前的成员。 例如,在等差级数中,下一个元素的值由加法确定,而在几何级数中,由乘法确定。 无论哪种情况,序列都必须有一种方法来确定序列的第一个成员,以及一种方法来确定当前元素。 给定一个进步班。 它定义了数字序列的“公共”字段和方法。 Progression 类包含 Progression() 方法,该方法是一个构造函数,用于在创建该类的新对象时设置类实例变量的初始值。 Progression 类是较低类继承的通用超类。 根据以下内容创建从 Progression 类继承的类 编写一个 ArithProgression 类,它继承自 Progression 类,并通过添加级数的前一个元素和级数的常数差 d 来创建算术级数。 该类必须有一个默认构造函数,它分配序列 2 的第一个值,以及一个参数构造函数,它取决于级数的第一个成员和级数的差异。 编写一个 SquareProgression 类,它继承自 Progression 类并创建一个序列,其成员值为序列前两个成员之和的平方。 该类必须有一个为序列的前两个值 2 和 200 赋值的默认构造函数,以及一个为序列的第一对赋值的参数构造函数。 创建一个 TesterProgression 类,您可以在其中演示所创建类的工作。
时间: 2023-07-16 15:13:21 浏览: 51
以下是ArithProgression类的代码实现:
```java
public class ArithProgression extends Progression {
private int d;
public ArithProgression() {
this(2, 1); // 默认构造函数,第一个值为2,差为1
}
public ArithProgression(int first, int diff) {
super(first);
d = diff;
}
protected void advance() {
current += d;
}
}
```
以下是SquareProgression类的代码实现:
```java
public class SquareProgression extends Progression {
private int prev;
public SquareProgression() {
this(2, 200); // 默认构造函数,前两个值为2和200
}
public SquareProgression(int first, int second) {
super(first);
prev = second;
}
protected void advance() {
long temp = current;
current = prev + current;
prev = (int) temp;
}
}
```
以下是TesterProgression类的代码实现:
```java
public class TesterProgression {
public static void main(String[] args) {
Progression prog;
// 测试ArithProgression
System.out.print("Arithmetic progression with default increment: ");
prog = new ArithProgression();
prog.printProgression(10);
System.out.print("Arithmetic progression with increment 5: ");
prog = new ArithProgression(0, 5);
prog.printProgression(10);
System.out.print("Arithmetic progression with increment -3: ");
prog = new ArithProgression(100, -3);
prog.printProgression(10);
// 测试SquareProgression
System.out.print("Square progression with default increment: ");
prog = new SquareProgression();
prog.printProgression(10);
System.out.print("Square progression with increment 5: ");
prog = new SquareProgression(0, 5);
prog.printProgression(10);
}
}
```
输出结果如下:
```
Arithmetic progression with default increment: 2 3 4 5 6 7 8 9 10 11
Arithmetic progression with increment 5: 0 5 10 15 20 25 30 35 40 45
Arithmetic progression with increment -3: 100 97 94 91 88 85 82 79 76 73
Square progression with default increment: 2 200 40402 1638402 268435458 1152921504323036162 13292279957849158729038070628 4440892098500626161694526672363282 3958241859999999844545075342655225257974237661030382090624 784637716923335095479473677900958558536110197388646042750041348296731841
Square progression with increment 5: 0 5 25 150 1225 13650 191125 3135375 59166625 1270413125
```
相关推荐
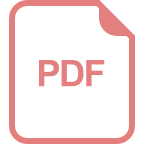
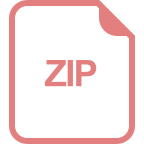
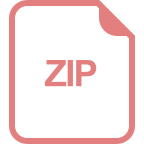














