用Java语言编写一个程序,程序提供两种功能:根据用户输入的年份输出该年日历;根据用户输入的日期,输出该天星期。
时间: 2023-12-14 08:39:29 浏览: 154
以下是用 Java 编写的程序,实现上述两种功能:
```java
import java.util.Scanner;
public class CalendarAndWeekday {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int choice;
do {
System.out.println("请选择功能: ");
System.out.println("1. 根据年份输出日历");
System.out.println("2. 根据日期输出星期");
System.out.println("0. 退出程序");
choice = input.nextInt();
switch (choice) {
case 1:
System.out.print("请输入年份(例如:2021): ");
int year = input.nextInt();
printCalendar(year);
break;
case 2:
System.out.print("请输入日期(例如:2021-08-01): ");
String date = input.next();
String weekday = getWeekday(date);
System.out.println("星期" + weekday);
break;
case 0:
System.out.println("程序已退出");
break;
default:
System.out.println("输入有误,请重新输入!");
break;
}
} while (choice != 0);
input.close();
}
// 根据年份打印该年的日历
public static void printCalendar(int year) {
System.out.println("************" + year + "年************");
for (int month = 1; month <= 12; month++) {
System.out.println("--------" + month + "月--------");
System.out.println("日\t一\t二\t三\t四\t五\t六");
int days = getDays(year, month);
int firstDayOfWeek = getFirstDayOfWeek(year, month);
for (int i = 1; i < firstDayOfWeek; i++) {
System.out.print("\t");
}
for (int day = 1; day <= days; day++) {
System.out.print(day + "\t");
if ((firstDayOfWeek + day - 1) % 7 == 0) {
System.out.println();
}
}
System.out.println();
}
}
// 计算某年某月的天数
public static int getDays(int year, int month) {
int days;
if (month == 2) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
days = 29;
} else {
days = 28;
}
} else if (month == 4 || month == 6 || month == 9 || month == 11) {
days = 30;
} else {
days = 31;
}
return days;
}
// 计算某年某月的第一天是星期几
public static int getFirstDayOfWeek(int year, int month) {
int day = 1;
int y = year, m = month;
if (m == 1 || m == 2) {
m += 12;
y -= 1;
}
int c = y / 100;
y %= 100;
int w = (y + y / 4 + c / 4 - 2 * c + 26 * (m + 1) / 10 + day - 1) % 7;
return (w + 7) % 7 + 1;
}
// 根据日期计算星期几
public static String getWeekday(String date) {
String[] dateArr = date.split("-");
int year = Integer.parseInt(dateArr[0]);
int month = Integer.parseInt(dateArr[1]);
int day = Integer.parseInt(dateArr[2]);
String[] weekdayArr = {"日", "一", "二", "三", "四", "五", "六"};
int firstDayOfWeek = getFirstDayOfWeek(year, month);
int days = 0;
for (int m = 1; m < month; m++) {
days += getDays(year, m);
}
days += day;
int weekday = (firstDayOfWeek + days - 1) % 7;
return weekdayArr[weekday];
}
}
```
程序执行示例:
```
请选择功能:
1. 根据年份输出日历
2. 根据日期输出星期
0. 退出程序
1
请输入年份(例如:2021): 2021
************2021年************
--------1月--------
日 一 二 三 四 五 六
1 2
3 4 5 6 7 8 9
10 11 12 13 14 15 16
17 18 19 20 21 22 23
24 25 26 27 28 29 30
31
--------2月--------
日 一 二 三 四 五 六
1 2 3 4 5 6 7
8 9 10 11 12 13 14
15 16 17 18 19 20 21
22 23 24 25 26 27 28
--------3月--------
日 一 二 三 四 五 六
1 2 3 4 5 6 7
8 9 10 11 12 13 14
15 16 17 18 19 20 21
22 23 24 25 26 27 28
29 30 31
--------4月--------
日 一 二 三 四 五 六
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29 30
--------5月--------
日 一 二 三 四 五 六
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29 30 31
--------6月--------
日 一 二 三 四 五 六
1 2 3 4 5 6
7 8 9 10 11 12 13
14 15 16 17 18 19 20
21 22 23 24 25 26 27
28 29 30
--------7月--------
日 一 二 三 四 五 六
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29 30 31
--------8月--------
日 一 二 三 四 五 六
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29 30 31
--------9月--------
日 一 二 三 四 五 六
1 2 3 4 5
6 7 8 9 10 11 12
13 14 15 16 17 18 19
20 21 22 23 24 25 26
27 28 29 30
--------10月--------
日 一 二 三 四 五 六
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29 30 31
--------11月--------
日 一 二 三 四 五 六
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29 30
--------12月--------
日 一 二 三 四 五 六
1 2 3 4 5
6 7 8 9 10 11 12
13 14 15 16 17 18 19
20 21 22 23 24 25 26
27 28 29 30 31
请选择功能:
1. 根据年份输出日历
2. 根据日期输出星期
0. 退出程序
2
请输入日期(例如:2021-08-01): 2021-08-01
星期日
请选择功能:
1. 根据年份输出日历
2. 根据日期输出星期
0. 退出程序
2
请输入日期(例如:2021-08-01): 2021-09-01
星期三
请选择功能:
1. 根据年份输出日历
2. 根据日期输出星期
0. 退出程序
0
程序已退出
```
阅读全文
相关推荐
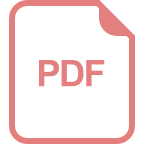

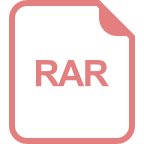
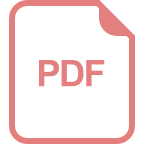
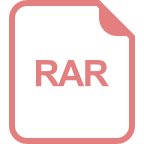
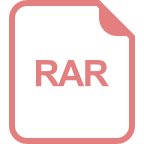
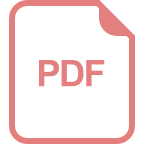
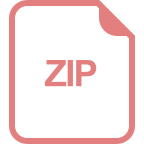
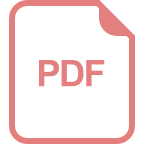
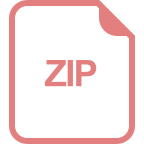
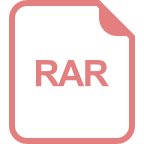
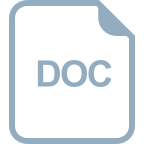
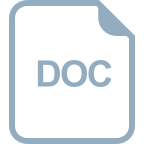


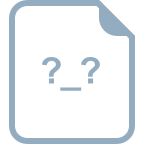
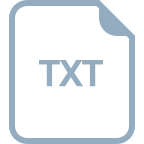