selenium by.xpath查找元素最新写法?
时间: 2023-11-01 20:28:21 浏览: 111
Selenium的`find_element_by_xpath`方法可以使用最新的XPath语法。以下是一些常用的XPath写法:
1. 通过元素的属性定位元素:
```python
driver.find_element_by_xpath("//tagname[@attribute='value']")
```
2. 通过元素的文本内容定位元素:
```python
driver.find_element_by_xpath("//tagname[contains(text(),'text')]")
```
3. 通过元素的子元素定位元素:
```python
driver.find_element_by_xpath("//parent_tagname/child_tagname")
```
4. 通过元素的兄弟元素定位元素:
```python
driver.find_element_by_xpath("//preceding-sibling::tagname")
driver.find_element_by_xpath("//following-sibling::tagname")
```
5. 通过元素的位置定位元素:
```python
driver.find_element_by_xpath("(//tagname)[position()=1]")
```
以上是一些常用的XPath写法,具体的语法可以根据实际情况进行调整。
相关问题
selenium如何通过by.xpath查找元素?
在 Selenium 中,可以使用 `find_element_by_xpath` 或 `find_elements_by_xpath` 方法通过 XPath 表达式查找元素。
例如,要通过 XPath 表达式 `//input[@id="example"]` 查找页面中 id 为 "example" 的输入框,可以使用以下代码:
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.example.com")
input_box = driver.find_element_by_xpath('//input[@id="example"]')
```
如果要查找多个匹配元素,可以使用 `find_elements_by_xpath` 方法,例如:
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.example.com")
elements = driver.find_elements_by_xpath('//div[@class="example"]')
```
这将查找页面中所有 class 为 "example" 的 div 元素,并返回一个元素列表。注意,`find_elements_by_xpath` 方法返回的是一个列表,即使只有一个匹配元素也是如此。 如果要访问列表中的特定元素,请使用索引。
举例说明selenium使用by_xpath查找元素
假设我们要查找一个网页中的“登录”按钮,我们可以通过以下步骤使用Selenium的by_xpath方法查找该元素:
1. 打开浏览器并访问网页:
```
from selenium import webdriver
# 创建浏览器对象
browser = webdriver.Chrome()
# 访问网页
browser.get('http://www.example.com')
```
2. 使用by_xpath方法查找元素:
```
# 使用by_xpath方法查找登录按钮
login_button = browser.find_element_by_xpath('//button[contains(text(), "登录")]')
```
其中,'//button'表示查找所有的button元素,'[contains(text(), "登录")]'表示其中包含文本内容为“登录”的元素。
3. 对查找到的元素进行操作:
```
# 点击登录按钮
login_button.click()
```
以上代码会在网页中查找包含文本内容为“登录”的按钮元素,并点击该按钮。
需要注意的是,XPath表达式中的单引号需要转义,所以最好使用双引号来表示字符串。另外,XPath表达式中的空格非常重要,要根据实际情况添加或省略。
阅读全文
相关推荐
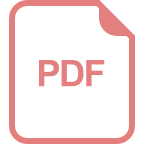
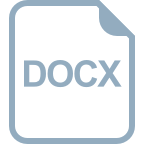
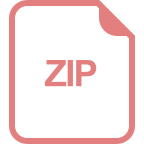
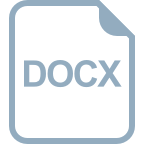


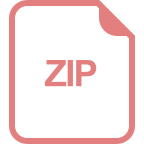
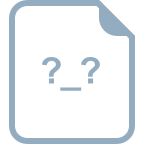
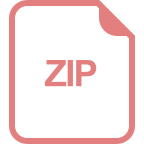
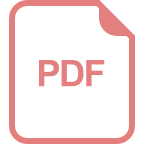
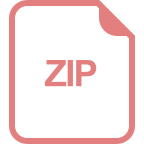
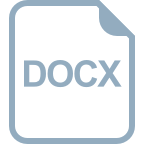

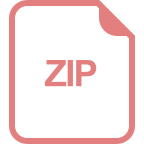
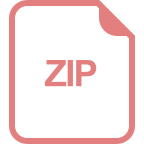