2.编写一个应用程序,用类描述计算机中CPU的速度和硬盘的容量,要求含有 四个类:PC、CPU、HardDisk和Test。
时间: 2024-02-28 13:55:14 浏览: 30
好的,下面是 Java 代码:
```java
// CPU 类
class CPU {
private double speed; // 速度
// 构造方法
public CPU(double speed) {
this.speed = speed;
}
// 获取速度
public double getSpeed() {
return speed;
}
}
// HardDisk 类
class HardDisk {
private int capacity; // 容量
// 构造方法
public HardDisk(int capacity) {
this.capacity = capacity;
}
// 获取容量
public int getCapacity() {
return capacity;
}
}
// PC 类
class PC {
private CPU cpu;
private HardDisk hardDisk;
// 构造方法,传入 CPU 和 HardDisk 对象
public PC(CPU cpu, HardDisk hardDisk) {
this.cpu = cpu;
this.hardDisk = hardDisk;
}
// 获取 CPU 速度
public double getCPUSpeed() {
return cpu.getSpeed();
}
// 获取硬盘容量
public int getHardDiskCapacity() {
return hardDisk.getCapacity();
}
}
// Test 类
public class Test {
public static void main(String[] args) {
// 创建 CPU 和 HardDisk 对象
CPU cpu = new CPU(2.8);
HardDisk hardDisk = new HardDisk(256);
// 创建 PC 对象
PC pc = new PC(cpu, hardDisk);
// 输出 CPU 速度和硬盘容量
System.out.println("CPU 速度为 " + pc.getCPUSpeed() + " GHz");
System.out.println("硬盘容量为 " + pc.getHardDiskCapacity() + " GB");
}
}
```
输出结果为:
```
CPU 速度为 2.8 GHz
硬盘容量为 256 GB
```
相关推荐
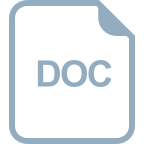
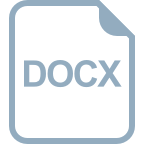
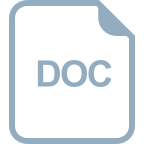














