生产一个学生选课管理系统
时间: 2023-12-18 12:03:41 浏览: 103
好的,一个学生选课管理系统一般包括以下几个模块:
1. 学生信息管理模块:包括学生基本信息的添加、修改、查询和删除等功能。
2. 课程信息管理模块:包括课程信息的添加、修改、查询和删除等功能。
3. 选课模块:学生可以通过选课模块进行课程的选取和退选。
4. 成绩管理模块:包括学生成绩的录入、修改和查询等功能。
5. 系统设置模块:包括管理员账号管理、权限设置等功能。
在实现这个系统时,可以采用Web开发技术,如使用Java、Python等语言结合SpringBoot、Django等框架进行开发。同时也可以使用前端开发技术,比如HTML、CSS、JavaScript等语言和Vue.js、React等框架进行开发。
需要考虑到系统的安全性,包括用户权限管理、数据加密等方面。同时,还需要考虑用户友好性和系统性能等因素,确保系统的稳定和高效运行。
相关问题
生产一个学生选课管理系统代码
好的,以下是一个简单的基于C++的学生选课管理系统代码示例。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Course {
private:
string name;
string id;
int capacity;
int enrolled;
public:
Course(string name, string id, int capacity) {
this->name = name;
this->id = id;
this->capacity = capacity;
this->enrolled = 0;
}
string get_name() {
return this->name;
}
string get_id() {
return this->id;
}
int get_capacity() {
return this->capacity;
}
int get_enrolled() {
return this->enrolled;
}
bool add_student() {
if (this->enrolled < this->capacity) {
this->enrolled++;
return true;
} else {
return false;
}
}
};
class Student {
private:
string name;
string id;
vector<Course*> courses;
public:
Student(string name, string id) {
this->name = name;
this->id = id;
}
string get_name() {
return this->name;
}
string get_id() {
return this->id;
}
void enroll(Course* course) {
if (course->add_student()) {
this->courses.push_back(course);
cout << "Enrolled " << course->get_name() << endl;
} else {
cout << "Course is full: " << course->get_name() << endl;
}
}
void drop(Course* course) {
bool found = false;
for (int i = 0; i < this->courses.size(); i++) {
if (this->courses[i]->get_id() == course->get_id()) {
this->courses.erase(this->courses.begin() + i);
course->drop_student();
cout << "Dropped " << course->get_name() << endl;
found = true;
break;
}
}
if (!found) {
cout << "Course not found: " << course->get_name() << endl;
}
}
void list_courses() {
cout << "Courses for " << this->name << ":" << endl;
for (int i = 0; i < this->courses.size(); i++) {
cout << "- " << this->courses[i]->get_name() << endl;
}
}
};
class CourseManager {
private:
vector<Course*> courses;
public:
void add_course() {
string name, id;
int capacity;
cout << "Enter course name: ";
cin >> name;
cout << "Enter course ID: ";
cin >> id;
cout << "Enter course capacity: ";
cin >> capacity;
courses.push_back(new Course(name, id, capacity));
}
void remove_course() {
string id;
cout << "Enter course ID: ";
cin >> id;
bool found = false;
for (int i = 0; i < courses.size(); i++) {
if (courses[i]->get_id() == id) {
courses.erase(courses.begin() + i);
found = true;
cout << "Course removed." << endl;
break;
}
}
if (!found) {
cout << "Course not found." << endl;
}
}
void list_courses() {
cout << "Course list:" << endl;
for (int i = 0; i < courses.size(); i++) {
Course* course = courses[i];
cout << "- " << course->get_name() << " (ID: " << course->get_id() << ") Capacity: " << course->get_capacity() << " Enrolled: " << course->get_enrolled() << endl;
}
}
Course* find_course(string id) {
for (int i = 0; i < courses.size(); i++) {
if (courses[i]->get_id() == id) {
return courses[i];
}
}
return NULL;
}
};
class StudentManager {
private:
vector<Student*> students;
public:
void add_student() {
string name, id;
cout << "Enter student name: ";
cin >> name;
cout << "Enter student ID: ";
cin >> id;
students.push_back(new Student(name, id));
}
void remove_student() {
string id;
cout << "Enter student ID: ";
cin >> id;
bool found = false;
for (int i = 0; i < students.size(); i++) {
if (students[i]->get_id() == id) {
students.erase(students.begin() + i);
found = true;
cout << "Student removed." << endl;
break;
}
}
if (!found) {
cout << "Student not found." << endl;
}
}
void list_students() {
cout << "Student list:" << endl;
for (int i = 0; i < students.size(); i++) {
Student* student = students[i];
cout << "- " << student->get_name() << " (ID: " << student->get_id() << ")" << endl;
student->list_courses();
}
}
Student* find_student(string id) {
for (int i = 0; i < students.size(); i++) {
if (students[i]->get_id() == id) {
return students[i];
}
}
return NULL;
}
};
int main() {
int choice;
CourseManager courseManager;
StudentManager studentManager;
while (true) {
cout << "Select an option:" << endl;
cout << "1. Add course" << endl;
cout << "2. Remove course" << endl;
cout << "3. List courses" << endl;
cout << "4. Add student" << endl;
cout << "5. Remove student" << endl;
cout << "6. List students" << endl;
cout << "7. Enroll student" << endl;
cout << "8. Drop student" << endl;
cout << "0. Exit" << endl;
cin >> choice;
switch (choice) {
case 1:
courseManager.add_course();
break;
case 2:
courseManager.remove_course();
break;
case 3:
courseManager.list_courses();
break;
case 4:
studentManager.add_student();
break;
case 5:
studentManager.remove_student();
break;
case 6:
studentManager.list_students();
break;
case 7: {
string studentId, courseId;
cout << "Enter student ID: ";
cin >> studentId;
Student* student = studentManager.find_student(studentId);
if (student == NULL) {
cout << "Student not found." << endl;
break;
}
cout << "Enter course ID: ";
cin >> courseId;
Course* course = courseManager.find_course(courseId);
if (course == NULL) {
cout << "Course not found." << endl;
break;
}
student->enroll(course);
break;
}
case 8: {
string studentId, courseId;
cout << "Enter student ID: ";
cin >> studentId;
Student* student = studentManager.find_student(studentId);
if (student == NULL) {
cout << "Student not found." << endl;
break;
}
cout << "Enter course ID: ";
cin >> courseId;
Course* course = courseManager.find_course(courseId);
if (course == NULL) {
cout << "Course not found." << endl;
break;
}
student->drop(course);
break;
}
case 0:
return 0;
default:
cout << "Invalid option." << endl;
break;
}
}
return 0;
}
```
该系统包括两个管理类:`CourseManager`和`StudentManager`,以及两个实体类:`Course`和`Student`。每个类都有一些方法,以实现相应的功能。
在主函数中,我们创建了`CourseManager`和`StudentManager`对象,并使用一个无限循环来等待用户输入选项。用户可以选择添加、删除、列出课程或学生,或者将学生注册到课程中,或将学生退课。系统会根据用户的选择调用相应的方法。
这只是一个简单的示例,实际的学生选课管理系统需要更多的功能和错误处理。但是,这应该可以帮助你开始编写自己的程序。
阅读全文
相关推荐
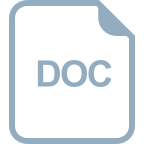
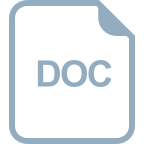
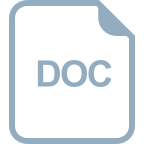
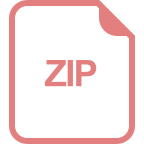
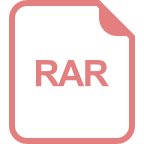
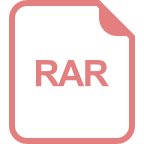
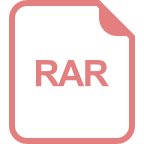
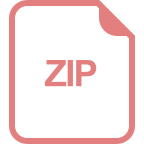
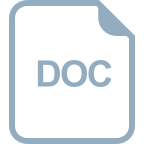
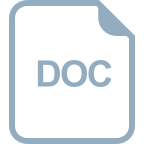
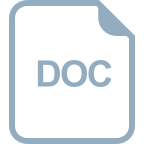
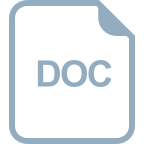
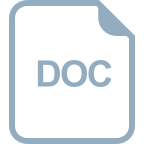
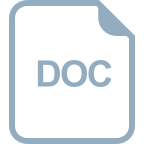
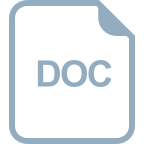
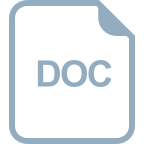
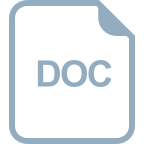
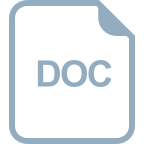