不同路径代码C++编译器且需要手动输入带ui界面
时间: 2024-01-14 13:03:24 浏览: 152
如果您需要在C++代码中添加UI界面来输入行数和列数,并计算不同路径问题,可以考虑使用以下方法:
1. 选择一个UI库或工具,例如Qt、wxWidgets等,来设计UI界面。您可以使用UI设计器来设计界面,并添加输入框和按钮等控件。
2. 将UI界面与C++代码进行连接,使用信号和槽机制来实现用户输入和计算结果的交互。您可以在按钮的槽函数中读取输入框的值,并将其传递给C++代码进行计算,然后在界面上显示计算结果。
3. 在C++代码中实现不同路径问题的计算。您可以使用动态分配的二维数组来保存状态,使用状态转移方程`dp[i][j] = dp[i-1][j] + dp[i][j-1]`,计算出每个位置的不同路径数。最后,返回右下角的值,即为从左上角到右下角的不同路径数。
下面是一个使用Qt库设计UI界面,并计算不同路径问题的C++代码示例:
```cpp
#include <QApplication>
#include <QWidget>
#include <QLabel>
#include <QLineEdit>
#include <QPushButton>
#include <QVBoxLayout>
#include <vector>
using namespace std;
class UniquePathsWindow : public QWidget {
public:
UniquePathsWindow(QWidget *parent = 0);
private slots:
void calculate(); // 计算槽函数
private:
QLabel *m_label1;
QLabel *m_label2;
QLineEdit *m_lineEdit1;
QLineEdit *m_lineEdit2;
QPushButton *m_button;
QLabel *m_resultLabel;
};
UniquePathsWindow::UniquePathsWindow(QWidget *parent)
: QWidget(parent)
{
m_label1 = new QLabel("行数:");
m_label2 = new QLabel("列数:");
m_lineEdit1 = new QLineEdit;
m_lineEdit2 = new QLineEdit;
m_button = new QPushButton("计算");
m_resultLabel = new QLabel;
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(m_label1);
layout->addWidget(m_lineEdit1);
layout->addWidget(m_label2);
layout->addWidget(m_lineEdit2);
layout->addWidget(m_button);
layout->addWidget(m_resultLabel);
setLayout(layout);
connect(m_button, SIGNAL(clicked()), this, SLOT(calculate())); // 连接信号和槽函数
}
void UniquePathsWindow::calculate()
{
int m = m_lineEdit1->text().toInt(); // 读取输入框的值
int n = m_lineEdit2->text().toInt();
vector<vector<int>> dp(m, vector<int>(n, 1)); // 初始化
for (int i = 1; i < m; i++) {
for (int j = 1; j < n; j++) {
dp[i][j] = dp[i-1][j] + dp[i][j-1]; // 状态转移方程
}
}
m_resultLabel->setText("从左上角到右下角的不同路径数为:" + QString::number(dp[m-1][n-1])); // 显示计算结果
}
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
UniquePathsWindow window;
window.setWindowTitle("不同路径问题");
window.show();
return app.exec();
}
```
上述代码使用Qt库来设计UI界面,包括输入框、按钮和结果显示标签等控件。在`calculate`槽函数中,读取输入框的值,并使用动态分配的二维数组来保存状态,计算出不同路径数。最后,在结果显示标签中显示计算结果。
在`main`函数中,创建`UniquePathsWindow`窗口实例,并显示窗口。您可以根据需要修改UI界面的样式和布局,以及计算的算法和UI交互方式。
这是一种使用Qt库设计UI界面,并计算不同路径问题的C++代码实现方式,它可以方便地进行UI设计和交互,但需要您熟悉Qt库和C++编程知识。
阅读全文
相关推荐
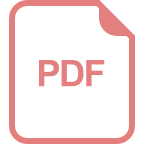




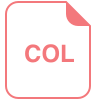
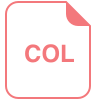
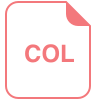
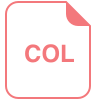
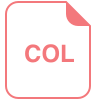
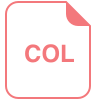
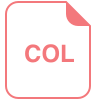
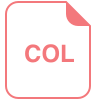
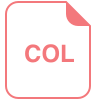
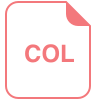
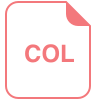
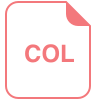