java pdf 图片压缩
时间: 2023-10-14 17:25:19 浏览: 56
可以使用 Java 的 iText 库来进行 PDF 图片压缩。以下是一个简单的示例代码:
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.Image;
import com.itextpdf.text.pdf.PdfWriter;
import com.itextpdf.text.pdf.PdfDictionary;
import com.itextpdf.text.pdf.PdfName;
import com.itextpdf.text.pdf.PdfObject;
import com.itextpdf.text.pdf.PdfArray;
import com.itextpdf.text.pdf.parser.PdfImageObject;
import java.io.FileOutputStream;
public class ImageCompressor {
public static void compressImage(String inputFilePath, String outputFilePath) {
try {
// 创建 PDF 文档对象并设置页面大小
Document document = new Document();
document.setPageSize(com.itextpdf.text.PageSize.A4);
// 创建 PDF Writer 对象并设置输出文件路径
PdfWriter.getInstance(document, new FileOutputStream(outputFilePath));
document.open();
// 加载图片并获取图像对象
Image image = Image.getInstance(inputFilePath);
PdfImageObject imageObject = new PdfImageObject(image, null);
// 获取原始图像的宽度和高度
int origWidth = imageObject.getWidth();
int origHeight = imageObject.getHeight();
// 计算新的图像宽度和高度
int newWidth = (int) (origWidth * 0.5);
int newHeight = (int) (origHeight * 0.5);
// 创建 PDF 图像对象并设置压缩参数
PdfDictionary imageDictionary = new PdfDictionary();
imageDictionary.put(PdfName.TYPE, PdfName.XOBJECT);
imageDictionary.put(PdfName.SUBTYPE, PdfName.IMAGE);
imageDictionary.put(PdfName.WIDTH, new PdfObject(newWidth));
imageDictionary.put(PdfName.HEIGHT, new PdfObject(newHeight));
imageDictionary.put(PdfName.BITSPERCOMPONENT, new PdfObject(imageObject.getBitsPerComponent()));
imageDictionary.put(PdfName.COLORSPACE, imageObject.getColorSpace());
imageDictionary.put(PdfName.FILTER, PdfName.DCTDECODE);
imageDictionary.put(PdfName.DECODEPARMS, new PdfArray(new int[] { 1, 0, 1, 0, 1, 0 }));
imageDictionary.put(PdfName.LENGTH, new PdfObject(imageObject.getStreamLength()));
// 将图像对象写入 PDF 文档
PdfWriter writer = document.getWriter();
PdfIndirectObject imageObjectRef = writer.addToBody(new PdfStream(imageObject.getStreamBytes()), false);
imageDictionary.put(PdfName.LENGTH, new PdfObject(imageObjectRef.getIndirectReference()));
writer.addToBody(imageDictionary, imageObjectRef.getIndirectReference());
document.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们将原始图像的大小减小了一半,并使用 JPEG 压缩算法将其写入 PDF 文档。您可以根据需要自定义压缩参数。
相关推荐
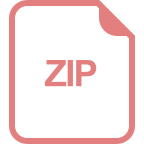
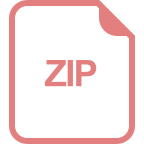
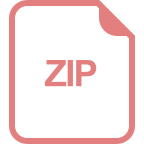














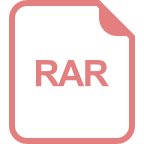