用Vue实现一个九宫格抽奖程序
时间: 2024-03-06 15:47:24 浏览: 101
好的,我可以帮你实现一个基于Vue的九宫格抽奖程序。以下是代码实现:
HTML部分:
```
<div id="app">
<div class="container">
<div class="grid" v-for="(item, index) in gridItems" :key="index" :class="{ active: item.active }" @click="handleClick(index)">
{{ item.prize }}
</div>
</div>
<div class="button" @click="handleStart">
{{ buttonText }}
</div>
</div>
```
CSS部分:
```
.container {
display: flex;
flex-wrap: wrap;
justify-content: center;
margin: 50px auto;
width: 500px;
}
.grid {
display: flex;
justify-content: center;
align-items: center;
width: 100px;
height: 100px;
border: 1px solid #ccc;
font-size: 24px;
cursor: pointer;
}
.grid.active {
background-color: #f00;
color: #fff;
}
.button {
display: flex;
justify-content: center;
align-items: center;
width: 200px;
height: 50px;
margin: 0 auto;
border: 1px solid #ccc;
font-size: 24px;
cursor: pointer;
}
```
JavaScript部分:
```
new Vue({
el: "#app",
data: {
gridItems: [
{ prize: "1", active: false },
{ prize: "2", active: false },
{ prize: "3", active: false },
{ prize: "4", active: false },
{ prize: "5", active: false },
{ prize: "6", active: false },
{ prize: "7", active: false },
{ prize: "8", active: false },
{ prize: "9", active: false }
],
buttonText: "开始抽奖",
timer: null,
index: 0,
count: 0
},
methods: {
handleClick(index) {
if (this.timer) return;
if (this.gridItems[index].active) return;
this.gridItems.forEach(item => (item.active = false));
this.gridItems[index].active = true;
},
handleStart() {
if (this.timer) return;
this.buttonText = "抽奖中...";
this.timer = setInterval(() => {
this.gridItems[this.index].active = false;
this.index = Math.floor(Math.random() * this.gridItems.length);
this.gridItems[this.index].active = true;
this.count++;
if (this.count > 20 && this.index === 0) {
clearInterval(this.timer);
this.timer = null;
this.count = 0;
this.buttonText = "再次抽奖";
alert("恭喜您获得1等奖!");
}
}, 100);
}
}
});
```
这个九宫格抽奖程序随机抽取一个格子,如果抽中的格子是第一个格子,则弹出恭喜获奖的提示框。可以根据自己的需求进行修改。
阅读全文
相关推荐
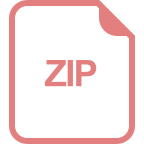

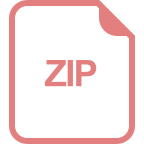
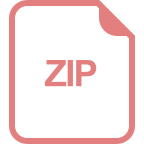
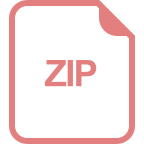
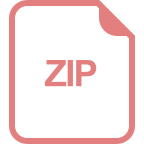