数据预处理使用Python,给出具体代码
时间: 2024-03-02 22:53:16 浏览: 27
好的,这里提供一份Python代码,用于从原始视频中提取帧并将其转换为张量:
```python
import cv2
import os
import numpy as np
import tensorflow as tf
# 路径参数
data_dir = 'path/to/dataset'
output_dir = 'path/to/output'
num_classes = 9
# 超参数
num_frames = 16
image_size = (224, 224)
# 获取所有视频文件
video_list = os.listdir(data_dir)
# 创建输出目录
if not os.path.exists(output_dir):
os.makedirs(output_dir)
# 加载模型,用于对帧进行处理
model = tf.keras.applications.resnet50.ResNet50(weights='imagenet', include_top=False)
# 遍历每个视频文件并提取帧
for video_file in video_list:
# 获取视频标签
label = int(video_file.split('_')[0]) - 1
label_onehot = tf.one_hot(label, num_classes)
# 打开视频文件
video_path = os.path.join(data_dir, video_file)
cap = cv2.VideoCapture(video_path)
frame_count = int(cap.get(cv2.CAP_PROP_FRAME_COUNT))
# 每隔若干帧提取一个样本
sample_indices = np.linspace(0, frame_count-1, num=num_frames, dtype=int)
for index in sample_indices:
# 读取帧并调整大小
cap.set(cv2.CAP_PROP_POS_FRAMES, index)
ret, frame = cap.read()
if not ret:
continue
frame = cv2.resize(frame, image_size)
# 对帧进行预处理
frame = tf.keras.applications.resnet50.preprocess_input(frame)
# 使用模型对帧进行特征提取,并将其转换为张量
features = model.predict(np.expand_dims(frame, axis=0))
features = tf.convert_to_tensor(features)
# 保存特征和标签
output_file = os.path.join(output_dir, f'{video_file}_{index:02d}.npz')
np.savez_compressed(output_file, features=features.numpy(), label=label_onehot.numpy())
cap.release()
```
这段代码假设您的原始视频数据集位于 `data_dir` 目录下,其中每个视频文件的名称以其标签开头,并以 `_` 符号分隔。例如,一个标签为1的视频文件可能被命名为 `1_video.mp4`。
代码将提取 `num_frames` 个帧,并将它们转换为形状为 `(num_frames, height, width, channels)` 的张量。每个张量都被保存为一个 `.npz` 文件,其中包含帧的特征和标签。
注意,这段代码使用了 `ResNet50` 模型来对帧进行特征提取。您可以根据需要更改模型,但请记得相应地调整预处理和特征提取步骤。
相关推荐
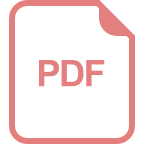














