java sftp 监控文件服务器文件变化
时间: 2023-08-05 11:46:16 浏览: 426
要在Java中监控SFTP服务器上的文件变化,可以使用JSch库。JSch是一个纯Java实现的SSH2协议的库,它提供了连接SSH服务器、进行文件传输、执行远程命令等功能。
下面是一个使用JSch监控SFTP服务器上的文件变化的示例代码:
```java
import com.jcraft.jsch.*;
public class SftpFileMonitor {
public static void main(String[] args) {
String host = "example.com"; // SFTP服务器地址
int port = 22; // SFTP服务器端口号
String username = "username"; // SFTP用户名
String password = "password"; // SFTP密码
String remoteFilePath = "/path/to/file"; // SFTP服务器上的文件路径
JSch jsch = new JSch();
try {
// 连接SFTP服务器
Session session = jsch.getSession(username, host, port);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
// 打开SFTP通道
ChannelSftp sftpChannel = (ChannelSftp) session.openChannel("sftp");
sftpChannel.connect();
// 监控文件变化
SftpMonitor monitor = new SftpMonitor(remoteFilePath);
sftpChannel.stat(remoteFilePath); // 确保文件存在
sftpChannel.addChannelSftpListener(monitor);
monitor.start();
// 等待文件变化
while (true) {
Thread.sleep(1000);
}
} catch (JSchException | InterruptedException | SftpException e) {
e.printStackTrace();
}
}
private static class SftpMonitor extends ChannelSftpAdapter implements Runnable {
private String remoteFilePath;
private Thread thread;
private boolean stopped;
public SftpMonitor(String remoteFilePath) {
this.remoteFilePath = remoteFilePath;
}
public void start() {
thread = new Thread(this);
thread.start();
}
public void stop() {
stopped = true;
}
@Override
public void run() {
while (!stopped) {
try {
Thread.sleep(5000); // 每5秒检查一次文件变化
SftpATTRS attrs = this.getStat(remoteFilePath);
long mtime = attrs.getMTime();
if (mtime != this.lastModified) {
this.lastModified = mtime;
System.out.println("File changed: " + remoteFilePath);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
private long lastModified = -1;
@Override
public void handle(ChannelSftp channel, int type, String message) {
if (type == ChannelSftp.SSH_FX_EOF) {
stop();
}
}
}
}
```
上述代码中,我们通过JSch连接SFTP服务器,并打开SFTP通道。然后,我们创建了一个SftpMonitor类来监控文件变化,它实现了ChannelSftpListener接口,可以监听文件上传、下载、删除等事件。SftpMonitor类中的run()方法会定时检查文件变化,如果文件的修改时间发生变化,则表示文件发生了变化。我们可以在控制台上输出文件变化的信息。
需要注意的是,SFTP服务器上的文件路径可以是相对路径或绝对路径,但必须是服务器上的路径。如果是相对路径,它是相对于当前用户的home目录。如果是绝对路径,它是相对于服务器的根目录。
阅读全文
相关推荐
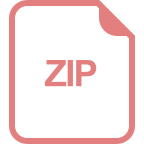
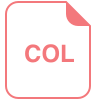
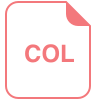
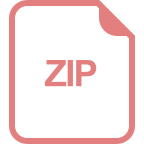
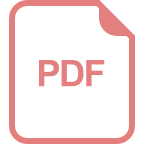
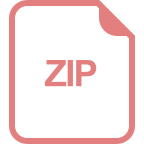
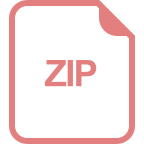
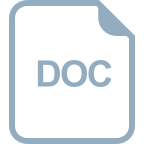
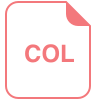
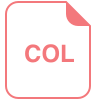
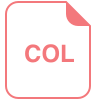
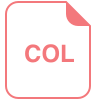
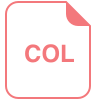
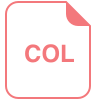
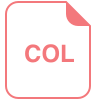
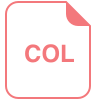