用c语言编写从数据文件读入若干整数值到数组,在数组中删除指定的数(该数键盘输入),处理后的数组元素再写入另一个数据文件。输出反馈,删除了几个数。
时间: 2024-04-30 15:23:00 浏览: 12
以下是一个可能的解答:
```c
#include <stdio.h>
#define MAX_SIZE 100
int main() {
// 打开数据文件
FILE *input_file = fopen("input.txt", "r");
if (input_file == NULL) {
printf("Failed to open input file.\n");
return 1;
}
// 读入整数值到数组
int array[MAX_SIZE];
int size = 0;
while (fscanf(input_file, "%d", &array[size]) == 1) {
size++;
}
// 关闭数据文件
fclose(input_file);
// 从键盘读入要删除的数
int to_delete;
printf("Enter the number to delete: ");
scanf("%d", &to_delete);
// 在数组中删除指定的数
int deleted_count = 0;
int i = 0;
while (i < size) {
if (array[i] == to_delete) {
size--;
deleted_count++;
} else {
array[i - deleted_count] = array[i];
}
i++;
}
// 打开输出文件
FILE *output_file = fopen("output.txt", "w");
if (output_file == NULL) {
printf("Failed to open output file.\n");
return 1;
}
// 将处理后的数组元素写入另一个数据文件
for (int i = 0; i < size; i++) {
fprintf(output_file, "%d ", array[i]);
}
// 关闭输出文件
fclose(output_file);
// 输出反馈
printf("Deleted %d numbers.\n", deleted_count);
return 0;
}
```
解释:
1. 首先打开输入数据文件,如果无法打开则输出错误信息并退出程序。
2. 在循环中使用 `fscanf` 读入整数值到数组,直到无法继续读入为止。每次成功读入后,数组的大小 `size` 增加 1。
3. 关闭输入数据文件。
4. 从键盘读入要删除的数。
5. 在循环中遍历数组,如果遇到要删除的数,则将数组的大小减 1,同时记录已经删除的数的数量。否则,将当前元素移动到正确位置。这里使用了一个 `deleted_count` 变量记录已经删除的数的数量,因为在移动元素时需要向前移动的距离取决于已经删除的数的数量。
6. 打开输出数据文件,如果无法打开则输出错误信息并退出程序。
7. 在循环中使用 `fprintf` 将处理后的数组元素写入输出数据文件。
8. 关闭输出数据文件。
9. 输出反馈,告诉用户删除了多少个数。
相关推荐
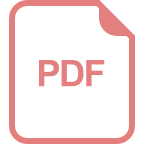
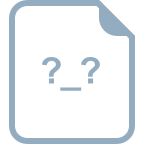














