基于在线评论的大规模群决策代码
时间: 2023-08-12 16:03:23 浏览: 220
基于在线评论的大规模群决策代码一般包括以下步骤:
1. 收集评论数据:从社交媒体、新闻网站或其他在线平台上收集大量评论数据,并将其存储在数据库或文本文件中。
2. 预处理数据:对收集到的评论数据进行预处理,包括去除停用词、词干提取、词向量表示等,以便于后续的分析。
3. 提取主题:使用主题模型等技术,对评论数据进行主题提取,以便于分析不同主题下的评论情感。
4. 分析情感:使用情感分析算法,对每个评论进行情感分析,得出其情感极性,如正面、负面、中性等。
5. 统计分析:对每个主题下的评论情感进行统计分析,得出每个主题下的情感分布情况。
6. 群体决策:根据统计分析结果,得出每个主题下的情感倾向,并作为群体决策的依据。
下面是一个基于在线评论的大规模群决策的代码示例:
```python
import pandas as pd
from nltk.sentiment import SentimentIntensityAnalyzer
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.decomposition import NMF
# 1. 收集评论数据
comment_data = pd.read_csv("comments.csv")
# 2. 预处理数据
vectorizer = TfidfVectorizer(stop_words="english")
X = vectorizer.fit_transform(comment_data["comment_text"])
# 3. 提取主题
nmf = NMF(n_components=10, random_state=1)
nmf.fit(X)
feature_names = vectorizer.get_feature_names()
# 4. 分析情感
sia = SentimentIntensityAnalyzer()
comment_data["sentiment"] = comment_data["comment_text"].apply(lambda x: sia.polarity_scores(x)["compound"])
# 5. 统计分析
topic_sentiments = []
for topic_idx, topic in enumerate(nmf.components_):
top_feature_indexes = topic.argsort()[:-10 - 1:-1]
top_features = [feature_names[i] for i in top_feature_indexes]
topic_sentiment = {"topic": topic_idx, "features": top_features, "sentiment": comment_data.groupby("topic")["sentiment"].mean()[topic_idx]}
topic_sentiments.append(topic_sentiment)
# 6. 群体决策
for topic_sentiment in topic_sentiments:
if topic_sentiment["sentiment"] > 0.5:
print("主题 %d 倾向于正面情感" % topic_sentiment["topic"])
elif topic_sentiment["sentiment"] < -0.5:
print("主题 %d 倾向于负面情感" % topic_sentiment["topic"])
else:
print("主题 %d 倾向于中性情感" % topic_sentiment["topic"])
```
以上代码中,我们使用了 `pandas` 库读取了一个名为 `comments.csv` 的评论数据文件,并使用 `NLTK` 库的情感分析算法对每个评论进行情感分析。然后,使用 `scikit-learn` 库的 `TfidfVectorizer` 和 `NMF` 算法对评论数据进行了主题提取,并统计了每个主题下的情感倾向。最后,根据统计分析结果进行了群体决策。
阅读全文
相关推荐
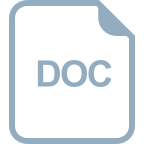
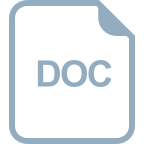
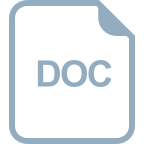
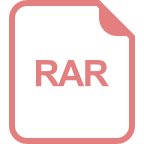
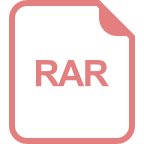
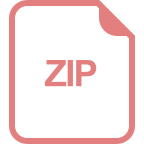
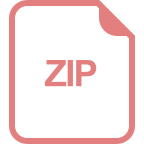
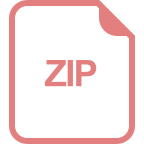
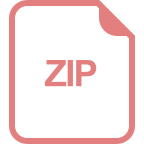
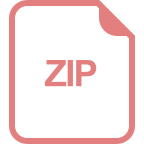
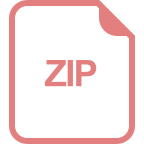
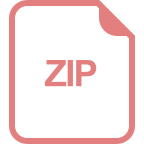
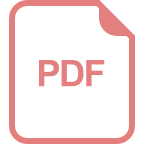
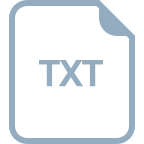
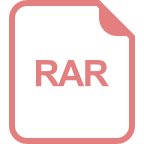
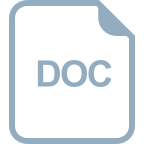
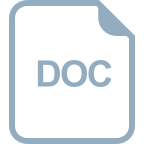
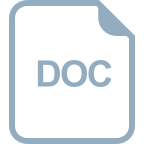
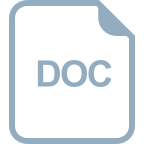