python 利用Pandas 对数据分箱,统计每个箱中的数量,使用matplotlib绘制出来
时间: 2023-12-06 13:44:49 浏览: 32
您好,关于您的问题,我可以为您提供一些思路和代码实现。首先,我们需要明确数据箱的方法,常见的有等距分箱和等频分箱,本次示例以等距分箱为例进行说明。
1. 数据准备
假设我们有一个 dataframe 包含某城市房价的数据,包括每个房子的面积(square_feet)和价格(price)。
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 创建模拟数据
np.random.seed(2022)
n = 200
df = pd.DataFrame({
'square_feet': np.random.normal(1200, 250, n),
'price': np.random.normal(600000, 90000, n)
})
```
2. 数据分箱
使用 Pandas 中的 cut 函数对数据进行分箱,此处等分为4组:
```python
# 将面积分为4组
df['square_feet_bins'] = pd.cut(df['square_feet'], bins=4)
# 统计每个箱子中的数量
square_feet_count = df.groupby('square_feet_bins')['square_feet'].count().reset_index()
```
3. 绘制图表
使用 matplotlib 中的 bar 函数绘制出每个箱子中的数据数量:
```python
# 绘制条形图
fig, ax = plt.subplots()
ax.bar(square_feet_count['square_feet_bins'], square_feet_count['square_feet'])
ax.set_xlabel('Square feet')
ax.set_ylabel('Count')
plt.show()
```
完整代码如下:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 创建模拟数据
np.random.seed(2022)
n = 200
df = pd.DataFrame({
'square_feet': np.random.normal(1200, 250, n),
'price': np.random.normal(600000, 90000, n)
})
# 将面积分为4组
df['square_feet_bins'] = pd.cut(df['square_feet'], bins=4)
# 统计每个箱子中的数量
square_feet_count = df.groupby('square_feet_bins')['square_feet'].count().reset_index()
# 绘制条形图
fig, ax = plt.subplots()
ax.bar(square_feet_count['square_feet_bins'], square_feet_count['square_feet'])
ax.set_xlabel('Square feet')
ax.set_ylabel('Count')
plt.show()
```
希望这个例子能对您有所帮助。
相关推荐
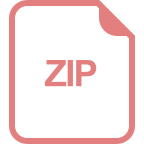
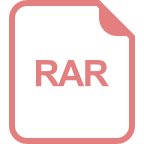
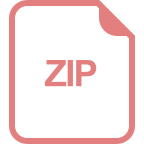














