void split_message(char* message, int message_len, int chunk_size) { int num_chunks = (message_len + chunk_size - 1) / chunk_size; for (int i = 0; i < num_chunks; i++) { char chunk[chunk_size + 1]; int start = i * chunk_size; int end = start + chunk_size; if (end > message_len) { end = message_len; } strncpy(chunk, message + start, end - start); chunk[end - start] = '\0'; printf("Chunk %d: %s\n", i + 1, chunk); } }
时间: 2024-04-03 09:34:01 浏览: 14
这段代码的作用是将一个给定长度的字符串(message)按照指定的块大小(chunk_size)分成多个块,并输出每个块的内容。具体地,代码首先计算出需要分成的块数(num_chunks),然后循环处理每个块。在循环中,代码首先根据块的编号计算出该块在字符串中的起始位置和终止位置(start和end),接着将该块的内容复制到一个名为chunk的字符数组中,并在chunk的末尾添加一个空字符,最后输出该块的内容。值得注意的是,当最后一个块的大小不足指定的块大小时,代码会将该块的大小设置为实际剩余字符的大小。
相关问题
num_chunk_samples = int(chunk_duration * sr)啥意思
这行代码的作用是计算每个音频块(chunk)的采样点数量。其中,chunk_duration是指定的音频块时长(以秒为单位),sr是音频的采样率(即每秒采样点数量)。通过将chunk_duration和sr相乘,可以得到每个音频块包含的采样点数量,但这是一个浮点数,需要使用int()将其转换成整数。最终,num_chunk_samples存储的是每个音频块的采样点数量。
stop = start + num_chunk_samples啥意思
这段代码是用来计算音频片段的结束位置的。
`start` 表示音频片段的起始位置,`num_chunk_samples` 表示想要提取的音频片段的采样数。那么 `start + num_chunk_samples` 就是这个音频片段的结束位置。
这样计算出音频片段的起始和结束位置后,就可以从原始音频文件中提取出这个片段,进行后续的处理和分析。
相关推荐
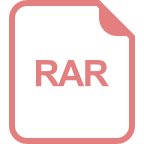
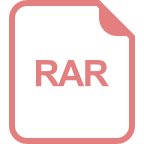












