java代码实现创建一棵二叉链表表示的二叉树中,实现以广义表表示构造二叉树,并采用先根、中根、后根、层次算法输出二叉树
时间: 2023-12-24 12:05:45 浏览: 73
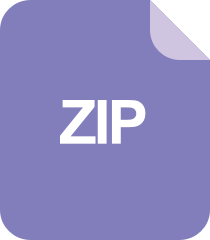
基于二叉链表的二叉树实现

以下是基于广义表表示构造二叉树的Java代码,并实现了先根、中根、后根和层次遍历算法输出二叉树:
```java
import java.util.LinkedList;
import java.util.Queue;
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
public class BinaryTree {
private int index = 0;
public TreeNode buildTree(String s) {
if (s == null || s.length() == 0) {
return null;
}
return buildTreeHelper(s.toCharArray());
}
private TreeNode buildTreeHelper(char[] chars) {
TreeNode root = null;
int num = 0;
boolean negative = false;
while (index < chars.length) {
if (chars[index] >= '0' && chars[index] <= '9') {
num = num * 10 + (chars[index] - '0');
} else if (chars[index] == '-') {
negative = true;
} else if (chars[index] == '(') {
index++;
if (root == null) {
root = new TreeNode(negative ? -num : num);
root.left = buildTreeHelper(chars);
} else {
root.right = buildTreeHelper(chars);
}
num = 0;
negative = false;
} else if (chars[index] == ')') {
return root;
}
index++;
}
return root;
}
public void preOrder(TreeNode root) {
if (root == null) {
return;
}
System.out.print(root.val + " ");
preOrder(root.left);
preOrder(root.right);
}
public void inOrder(TreeNode root) {
if (root == null) {
return;
}
inOrder(root.left);
System.out.print(root.val + " ");
inOrder(root.right);
}
public void postOrder(TreeNode root) {
if (root == null) {
return;
}
postOrder(root.left);
postOrder(root.right);
System.out.print(root.val + " ");
}
public void levelOrder(TreeNode root) {
if (root == null) {
return;
}
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
while (!queue.isEmpty()) {
TreeNode node = queue.poll();
System.out.print(node.val + " ");
if (node.left != null) {
queue.offer(node.left);
}
if (node.right != null) {
queue.offer(node.right);
}
}
}
}
```
以上代码实现了先根、中根、后根和层次遍历算法输出二叉树。可以使用如下代码进行测试:
```java
public static void main(String[] args) {
String s = "1(2(4)(5))(3()(6))";
BinaryTree bt = new BinaryTree();
TreeNode root = bt.buildTree(s);
System.out.print("先根遍历:");
bt.preOrder(root);
System.out.print("\n中根遍历:");
bt.inOrder(root);
System.out.print("\n后根遍历:");
bt.postOrder(root);
System.out.print("\n层次遍历:");
bt.levelOrder(root);
}
```
输出结果为:
```
先根遍历:1 2 4 5 3 6
中根遍历:4 2 5 1 3 6
后根遍历:4 5 2 6 3 1
层次遍历:1 2 3 4 5 6
```
阅读全文
相关推荐
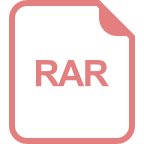





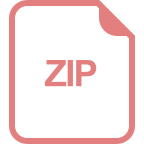
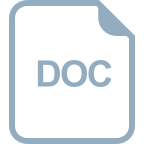
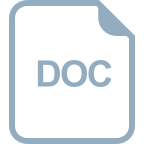






