openssl对称加密示例代码
时间: 2023-07-05 22:08:04 浏览: 49
下面是使用 OpenSSL 实现对称加密的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/evp.h>
#define BLOCK_SIZE 16
int main(int argc, char **argv) {
// 明文和密钥
const unsigned char *plaintext = (const unsigned char *)"This is a message.";
const unsigned char *key = (const unsigned char *)"This is a key.";
// 分配输出缓冲区
unsigned char *ciphertext = (unsigned char *)malloc(strlen(plaintext) + BLOCK_SIZE);
memset(ciphertext, 0, strlen(plaintext) + BLOCK_SIZE);
// 创建并初始化上下文
EVP_CIPHER_CTX *ctx = EVP_CIPHER_CTX_new();
EVP_CIPHER_CTX_init(ctx);
// 设置加密算法和密钥
const EVP_CIPHER *cipher = EVP_aes_128_ecb(); // 使用 AES-128 ECB 模式
EVP_EncryptInit_ex(ctx, cipher, NULL, key, NULL);
// 加密数据
int ciphertext_len = 0;
EVP_EncryptUpdate(ctx, ciphertext, &ciphertext_len, plaintext, strlen(plaintext));
// 结束加密过程,输出最后一块密文
int final_len = 0;
EVP_EncryptFinal_ex(ctx, ciphertext + ciphertext_len, &final_len);
ciphertext_len += final_len;
// 输出密文
printf("Ciphertext: ");
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
// 释放资源
EVP_CIPHER_CTX_free(ctx);
free(ciphertext);
return 0;
}
```
上面的代码使用 AES-128 ECB 模式加密明文数据,并使用密钥对数据进行加密。在实际使用中,应该根据需要选择合适的加密算法和加密模式,并采取适当的密钥管理措施来保证数据安全。
相关推荐
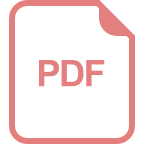
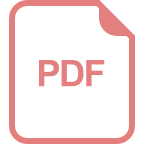
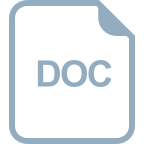














