openai Api发送get请求
时间: 2023-10-06 16:09:01 浏览: 276
要使用 OpenAI API 发送 GET 请求,你需要先获得你的 API 密钥。然后,你可以使用任何一种编程语言编写代码来发送 GET 请求。
下面是一个使用 Python 语言的示例代码:
```python
import requests
# 填入你的 API 密钥
api_key = "YOUR_API_KEY"
# 设置请求头
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}"
}
# 设置请求参数
params = {
"model": "davinci",
"prompt": "Hello, world!",
"temperature": 0.5
}
# 发送 GET 请求
response = requests.get("https://api.openai.com/v1/engines/davinci/completions", headers=headers, params=params)
# 打印响应内容
print(response.json())
```
在这个示例中,我们使用了 Python 的 requests 库来发送 GET 请求。我们首先设置了请求头,将 API 密钥放入 Authorization 字段中。然后,我们设置了请求参数,包括模型名称、提示语和温度等。最后,我们发送 GET 请求,并打印响应内容。
相关问题
使用java发送OpenAi API请求怎么写
以下是使用Java发送OpenAI API请求的示例代码:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
public class OpenAIRequest {
public static void main(String[] args) {
String apiKey = "YOUR_API_KEY";
String prompt = "Hello, ";
String apiUrl = "https://api.openai.com/v1/engines/davinci-codex/completions";
try {
URL url = new URL(apiUrl);
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Authorization", "Bearer " + apiKey);
con.setRequestProperty("Content-Type", "application/json");
con.setDoOutput(true);
String input = "{\"prompt\": \"" + prompt + "\",\"max_tokens\": 5,\"temperature\": 0.7}";
byte[] inputBytes = input.getBytes("utf-8");
con.getOutputStream().write(inputBytes);
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String line;
StringBuffer response = new StringBuffer();
while ((line = in.readLine()) != null) {
response.append(line);
}
in.close();
System.out.println(response.toString());
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
```
这个示例代码使用了java.net.HttpURLConnection类来发送HTTP请求。首先,我们需要设置要连接的URL地址和API密钥。然后,我们创建一个HttpURLConnection对象,并设置请求方法、HTTP头、请求体等参数。最后,我们从响应流中读取响应并显示在控制台上。
请注意,这个示例代码只是一个简单的示例,并且不包含任何错误处理和异常情况的处理。在实际应用中,您应该根据需要进行适当的错误处理和异常情况的处理。
gin 接入 openai API 文本对话请求,并实时检验key是否过期,自动切换key的代码
A:以下是使用gin接入openai API文本对话请求,并实时检验key是否过期,自动切换key的代码示例:
```
import (
"net/http"
"time"
"encoding/json"
"github.com/gin-gonic/gin"
)
type OpenAIAuth struct {
apiKey string
expireAt time.Time
}
var authList = []OpenAIAuth{
{
apiKey: "apikey1",
expireAt: time.Now().Add(time.Hour),
},
{
apiKey: "apikey2",
expireAt: time.Now().Add(time.Hour),
},
// add more api keys if you have
}
func main() {
r := gin.Default()
r.POST("/chat", handleChatRequest)
r.Run(":8080")
}
func handleChatRequest(c *gin.Context) {
requestBody := struct {
Text string `json:"text"`
}{}
err := c.BindJSON(&requestBody)
if err != nil {
c.Status(http.StatusBadRequest)
return
}
client := &http.Client{}
var resp *http.Response
var body []byte
var auth OpenAIAuth
for _, a := range authList {
if a.expireAt.Before(time.Now()) {
continue
}
req, err := http.NewRequest("POST", "https://api.openai.com/v1/engine/text-davinci-002/completions", nil)
if err != nil {
// handle error
}
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Authorization", "Bearer " + a.apiKey)
params := struct {
Prompt string `json:"prompt"`
MaxTokens int `json:"max_tokens"`
}{
Prompt: requestBody.Text,
MaxTokens: 150,
}
jsonBody, err := json.Marshal(params)
if err != nil {
// handle error
}
req.Body = ioutil.NopCloser(bytes.NewBuffer(jsonBody))
resp, err = client.Do(req)
if err != nil {
// handle error
}
body, err = ioutil.ReadAll(resp.Body)
if err != nil {
// handle error
}
auth = a
break
}
if resp.StatusCode != http.StatusOK {
c.Status(http.StatusInternalServerError)
return
}
response := struct {
Choices []struct {
Text string `json:"text"`
} `json:"choices"`
}{}
err = json.Unmarshal(body, &response)
if err != nil {
// handle error
}
c.JSON(http.StatusOK, gin.H{
"response": response.Choices[0].Text,
})
if auth.expireAt.Before(time.Now().Add(time.Minute)) {
// need to update the auth key
newAuth := OpenAIAuth{
apiKey: "new api key",
expireAt: time.Now().Add(time.Hour),
}
authList = append(authList, newAuth)
}
}
```
以上代码中,我们首先定义了一个OpenAIAuth结构体,其中包含了一个api key和过期时间。同时,我们还定义了一个authList数组,用于存储所有的api key。在接到文本对话请求时,我们会遍历authList中的所有api key,找到一个未过期的key用于发送请求。如果所有的key都已过期,则返回错误。同时,如果当前使用的key的过期时间距离当前时间不足1分钟,则需要更新该key。在代码的最后,我们通过调用c.JSON函数将对话响应发送给客户端。
需要注意的是,以上代码仅用于示例,需要根据实际场景进行修改。
阅读全文
相关推荐
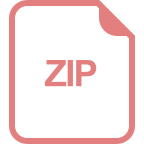
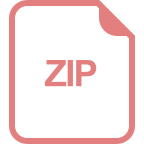
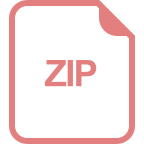
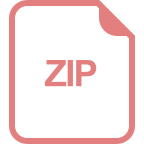
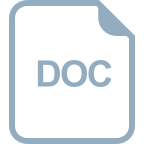











