vue3 ts写cnode
时间: 2024-05-12 10:08:27 浏览: 7
好的,下面我给你一个简单的示例,演示如何使用Vue3和TypeScript编写CNode社区的前端。
首先,你需要安装Vue CLI,可以通过以下命令进行安装:
```
npm install -g @vue/cli
```
接下来,创建一个新的Vue项目:
```
vue create my-cnode-app
```
在创建项目时,选择手动选择特性,勾选TypeScript。
然后,安装以下依赖:
```
npm install vue-router axios
npm install --save-dev @types/vue @types/vue-router @types/axios
```
这些依赖将帮助我们实现路由和HTTP请求。
接下来,在`src`文件夹下创建以下文件:
- `components/TopicList.vue`
- `components/TopicDetail.vue`
- `router/index.ts`
- `api/index.ts`
- `types/index.ts`
在`TopicList.vue`中,我们可以使用以下代码获取CNode社区主题列表:
```html
<template>
<div>
<h1>Topic List</h1>
<ul>
<li v-for="topic in topics" :key="topic.id">
<router-link :to="{ name: 'topic', params: { id: topic.id } }">{{ topic.title }}</router-link>
</li>
</ul>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
import { getTopics } from '@/api';
import { Topic } from '@/types';
export default defineComponent({
name: 'TopicList',
setup() {
const topics = ref<Topic[]>([]);
getTopics().then((res) => {
topics.value = res.data;
});
return {
topics,
};
},
});
</script>
```
在`TopicDetail.vue`中,我们可以使用以下代码获取CNode社区主题详情:
```html
<template>
<div>
<h1>{{ topic?.title }}</h1>
<p v-html="topic?.content"></p>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
import { getTopic } from '@/api';
import { Topic } from '@/types';
export default defineComponent({
name: 'TopicDetail',
setup(props) {
const topicId = ref(props.route.params.id);
const topic = ref<Topic>();
getTopic(topicId.value).then((res) => {
topic.value = res.data;
});
return {
topic,
};
},
});
</script>
```
在`router/index.ts`中,我们可以使用以下代码定义路由:
```ts
import { createRouter, createWebHistory } from 'vue-router';
import TopicList from '@/components/TopicList.vue';
import TopicDetail from '@/components/TopicDetail.vue';
const routes = [
{
path: '/',
name: 'home',
component: TopicList,
},
{
path: '/topic/:id',
name: 'topic',
component: TopicDetail,
},
];
const router = createRouter({
history: createWebHistory(),
routes,
});
export default router;
```
在`api/index.ts`中,我们可以使用以下代码定义与CNode社区API交互的函数:
```ts
import axios from 'axios';
import { Topic } from '@/types';
const api = axios.create({
baseURL: 'https://cnodejs.org/api/v1',
});
export function getTopics(): Promise<{ data: Topic[] }> {
return api.get('/topics');
}
export function getTopic(id: string): Promise<{ data: Topic }> {
return api.get(`/topic/${id}`);
}
```
在`types/index.ts`中,我们可以使用以下代码定义与CNode社区API交互的数据类型:
```ts
export interface Topic {
id: string;
title: string;
content: string;
}
```
最后,在`main.ts`中使用以下代码引入路由和根组件:
```ts
import { createApp } from 'vue';
import router from '@/router';
import App from './App.vue';
createApp(App).use(router).mount('#app');
```
这就是一个简单的Vue3 TypeScript应用程序,用于获取CNode社区的主题列表和主题详情。你可以根据自己的需求进行更改和扩展。
相关推荐
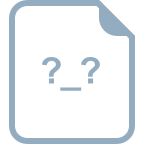














