Linux下实现定时器
时间: 2023-11-30 12:57:42 浏览: 94
在Linux中,有几种方法可以实现定时器功能:
1. 使用系统调用 alarm():该调用会在指定的时间(以秒为单位)后触发SIGALRM信号。可以通过注册信号处理函数来处理该信号,实现定时器功能。例如:
```
#include <unistd.h>
#include <signal.h>
void handler(int signum) {
// 定时器触发后的处理逻辑
}
int main() {
signal(SIGALRM, handler);
alarm(5); // 5秒后触发SIGALRM信号
while (1) {} // 持续运行程序,直到收到SIGALRM信号
return 0;
}
```
2. 使用timer_create()函数:该函数可以创建一个定时器,精度可以达到毫秒级别。例如:
```
#include <time.h>
#include <signal.h>
void handler(int signum) {
// 定时器触发后的处理逻辑
}
int main() {
struct sigevent sev;
timer_t timerid;
struct itimerspec its;
// 创建定时器
sev.sigev_notify = SIGEV_SIGNAL;
sev.sigev_signo = SIGUSR1;
sev.sigev_value.sival_ptr = &timerid;
timer_create(CLOCK_REALTIME, &sev, &timerid);
// 设置定时器
its.it_value.tv_sec = 1;
its.it_value.tv_nsec = 0;
its.it_interval.tv_sec = 1;
its.it_interval.tv_nsec = 0;
timer_settime(timerid, 0, &its, NULL);
// 注册信号处理函数
signal(SIGUSR1, handler);
while (1) {} // 持续运行程序,直到收到SIGUSR1信号
return 0;
}
```
3. 使用select()函数:该函数可以等待多个文件描述符中的任意一个就绪,可以通过设置超时时间实现定时器功能。例如:
```
#include <stdio.h>
#include <sys/select.h>
int main() {
fd_set rfds;
struct timeval tv;
while (1) {
// 清空文件描述符集合
FD_ZERO(&rfds);
// 设置需要监视的文件描述符
FD_SET(0, &rfds); // 监视标准输入
// 设置超时时间
tv.tv_sec = 5;
tv.tv_usec = 0;
// 等待文件描述符就绪或超时
int ret = select(1, &rfds, NULL, NULL, &tv);
if (ret == -1) {
perror("select");
} else if (ret == 0) {
printf("Timeout\n"); // 超时处理逻辑
} else {
if (FD_ISSET(0, &rfds)) {
printf("Input available\n"); // 文件描述符就绪处理逻辑
}
}
}
return 0;
}
```
这些方法都可以实现定时器功能,选择哪种方法取决于具体的场景和需求。
阅读全文
相关推荐
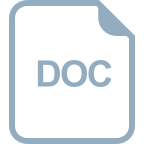
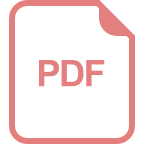
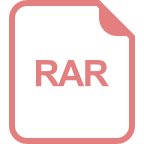
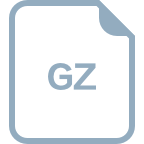
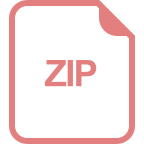
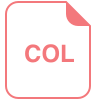


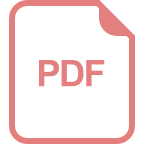
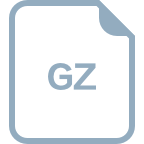
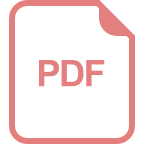
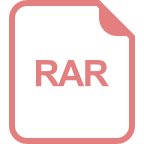
