用c++编写显示某年某月某日是星期几的代码,已知1980年1月1日 是星期二
时间: 2023-05-29 18:07:41 浏览: 416
以下是用C语言编写的代码:
```c
#include <stdio.h>
int main() {
int year, month, day;
printf("请输入年份:");
scanf("%d", &year);
printf("请输入月份:");
scanf("%d", &month);
printf("请输入日期:");
scanf("%d", &day);
int days[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int total_days = 0;
for (int i = 1980; i < year; i++) {
if (i % 4 == 0 && i % 100 != 0 || i % 400 == 0) {
total_days += 366;
} else {
total_days += 365;
}
}
for (int i = 0; i < month - 1; i++) {
total_days += days[i];
if (i == 1 && (year % 4 == 0 && year % 100 != 0 || year % 400 == 0)) {
total_days += 1; // 闰年2月加1天
}
}
total_days += day - 1; // 当月天数要减1才能计算
int week_day = (total_days + 2) % 7; // 1980年1月1日是星期二
char* week_days[] = {"星期一", "星期二", "星期三", "星期四", "星期五", "星期六", "星期日"};
printf("%d年%d月%d日是%s\n", year, month, day, week_days[week_day]);
return 0;
}
```
该程序通过输入年份、月份和日期,计算出这一天是星期几。具体思路为:
1. 定义一个整型数组days,表示每个月的天数。
2. 通过循环累加出输入年份之前的总天数。
3. 再通过循环累加出输入月份之前的总天数。
4. 最后加上输入日期,得到总共的天数。
5. 用总天数加上1980年1月1日是星期二的偏移量,再取模7,即可得到这一天是星期几。
6. 最后输出结果,使用char*类型的数组week_days存储星期几的字符串,根据计算结果输出相应的字符串即可。
需要注意的是,闰年2月有29天,需要特判。另外,当月天数要减1才能计算。
阅读全文
相关推荐
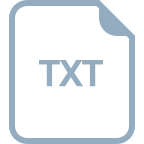


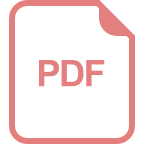

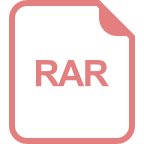
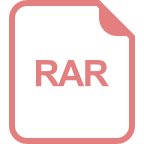
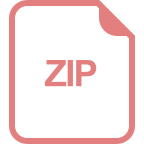
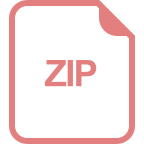
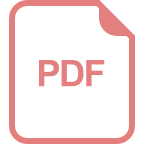