xml解析实体类怎么格式化呢
时间: 2024-03-10 22:47:05 浏览: 99
在 C# 中,可以使用 XmlSerializer 类将 XML 数据反序列化为实体类对象。具体的代码如下:
假设我们有一个如下的 XML 文件:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<book>
<title>Harry Potter and the Philosopher's Stone</title>
<author>J.K. Rowling</author>
<price>19.99</price>
</book>
```
那么我们可以定义一个 Book 类来表示这个 XML 文件中的数据:
```csharp
public class Book
{
public string Title { get; set; }
public string Author { get; set; }
public decimal Price { get; set; }
}
```
接着,我们可以使用 XmlSerializer 类将 XML 数据反序列化为 Book 对象:
```csharp
string xmlString = "<?xml version=\"1.0\" encoding=\"UTF-8\"?><book><title>Harry Potter and the Philosopher's Stone</title><author>J.K. Rowling</author><price>19.99</price></book>";
XmlSerializer serializer = new XmlSerializer(typeof(Book));
using (StringReader reader = new StringReader(xmlString))
{
Book book = (Book)serializer.Deserialize(reader);
Console.WriteLine($"Title: {book.Title}, Author: {book.Author}, Price: {book.Price}");
}
```
在上面的代码中,我们首先定义了 Book 类来表示 XML 文件中的数据。接着,我们将 XML 数据保存到字符串变量 xmlString 中,然后使用 XmlSerializer 类将其反序列化为 Book 对象。最后,我们输出 Book 对象的属性值。
需要注意的是,如果 XML 数据中的日期格式不符合 AllXsd datetime 格式,那么反序列化时会出现异常。因此,在定义实体类时,需要确保日期属性的类型是 DateTime 类型,并且在反序列化时使用 XmlConvert.ToDateTime 方法将日期字符串转换为 DateTime 对象。例如:
```csharp
public class Book
{
public string Title { get; set; }
public string Author { get; set; }
public decimal Price { get; set; }
public DateTime PublishDate { get; set; }
}
string xmlString = "<?xml version=\"1.0\" encoding=\"UTF-8\"?><book><title>Harry Potter and the Philosopher's Stone</title><author>J.K. Rowling</author><price>19.99</price><publishDate>2013-04-19T14:33:26</publishDate></book>";
XmlSerializer serializer = new XmlSerializer(typeof(Book));
using (StringReader reader = new StringReader(xmlString))
{
Book book = (Book)serializer.Deserialize(reader);
Console.WriteLine($"Title: {book.Title}, Author: {book.Author}, Price: {book.Price}, PublishDate: {book.PublishDate}");
}
```
在上面的代码中,我们新增了一个 PublishDate 属性来表示 XML 文件中的日期数据,并且在 XML 文件中使用了 AllXsd datetime 格式。在反序列化时,我们可以直接将日期字符串转换为 DateTime 对象。
阅读全文
相关推荐
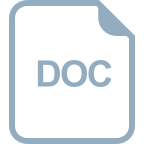
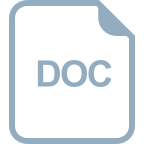
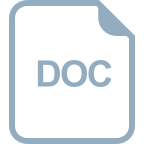
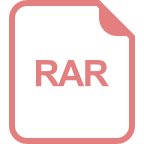
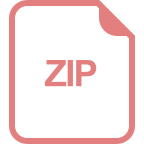
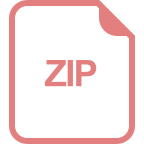
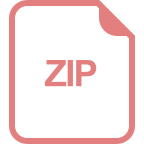
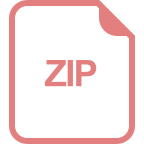
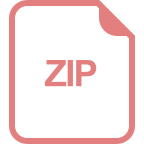
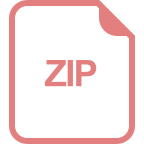
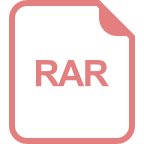
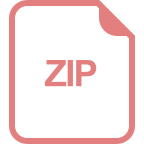
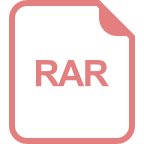
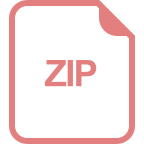
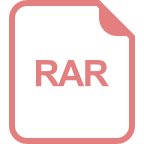
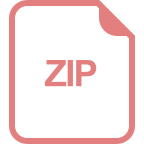
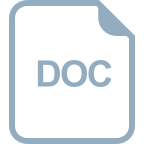
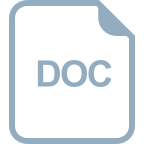
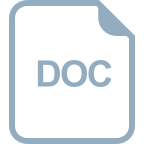