C语言编程实现通讯簿单向链表建立、删除、插入、输出
时间: 2023-09-28 12:10:18 浏览: 83
下面是一个简单的C语言程序,通过单向链表实现通讯簿的建立、删除、插入和输出。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义通讯录结构体
typedef struct contact {
char name[20]; // 姓名
char phone[20]; // 电话
struct contact *next; // 指向下一个节点的指针
}Contact;
// 创建新节点
Contact *createNode(char *name, char *phone) {
Contact *newNode = (Contact *)malloc(sizeof(Contact));
strcpy(newNode->name, name);
strcpy(newNode->phone, phone);
newNode->next = NULL;
return newNode;
}
// 插入节点
void insertNode(Contact **head, char *name, char *phone) {
Contact *newNode = createNode(name, phone);
if (*head == NULL) {
// 链表为空,直接插入
*head = newNode;
} else {
// 链表不为空,遍历到尾部插入
Contact *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = newNode;
}
}
// 删除节点
void deleteNode(Contact **head, char *name) {
if (*head == NULL) {
printf("通讯录为空,无法删除!\n");
return;
}
Contact *p = *head;
Contact *q = NULL;
while (p != NULL && strcmp(p->name, name) != 0) {
q = p;
p = p->next;
}
if (p == NULL) {
printf("通讯录中没有此人!\n");
return;
}
if (q == NULL) {
// 删除的是头节点
*head = p->next;
} else {
// 删除的是中间节点或尾节点
q->next = p->next;
}
free(p);
}
// 输出通讯录
void printList(Contact *head) {
if (head == NULL) {
printf("通讯录为空!\n");
return;
}
Contact *p = head;
printf("通讯录如下:\n");
while (p != NULL) {
printf("姓名:%s,电话:%s\n", p->name, p->phone);
p = p->next;
}
}
int main() {
Contact *head = NULL;
int choice;
char name[20], phone[20];
while (1) {
printf("1. 添加联系人\n");
printf("2. 删除联系人\n");
printf("3. 显示通讯录\n");
printf("4. 退出程序\n");
printf("请输入你的选择:");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入姓名:");
scanf("%s", name);
printf("请输入电话:");
scanf("%s", phone);
insertNode(&head, name, phone);
break;
case 2:
printf("请输入要删除的联系人姓名:");
scanf("%s", name);
deleteNode(&head, name);
break;
case 3:
printList(head);
break;
case 4:
exit(0);
default:
printf("输入有误,请重新输入!\n");
break;
}
}
return 0;
}
```
以上程序实现了通讯簿的单向链表建立、删除、插入和输出。用户可以根据提示操作通讯簿,可以添加联系人、删除联系人和显示通讯录。
阅读全文
相关推荐
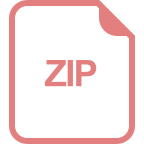
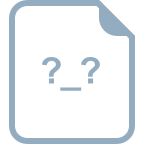
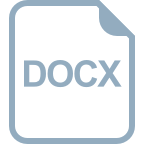
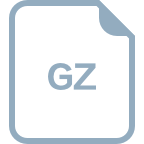
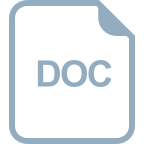
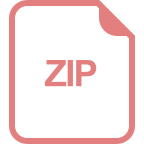
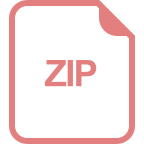
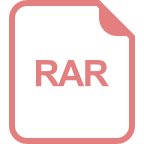
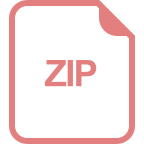
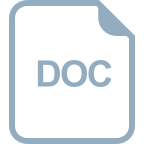