用python 写一个遗传算法的代码
时间: 2023-08-31 20:22:32 浏览: 107
### 回答1:
答:首先,要写一个遗传算法的代码,需要使用Python语言。首先,需要定义一个适合遗传算法的问题,以及遗传算法的参数,如种群大小、交叉概率和变异概率等。接下来,需要定义种群,并且初始化每个个体的基因,然后开始迭代,包括计算适应度、选择、交叉和变异等步骤,直到满足停止条件为止。
### 回答2:
遗传算法是一种优化问题的求解方法,它基于生物进化的思想,通过模拟自然选择、交叉和变异等基本操作,对个体进行进化和优化。下面是用Python编写的遗传算法代码的一个简单示例:
```python
import random
# 设置种群大小
population_size = 100
# 设置染色体长度
chromosome_length = 50
# 设置迭代次数
max_generations = 500
# 设置突变概率
mutation_rate = 0.01
# 初始化种群
def initialize_population():
population = []
for i in range(population_size):
chromosome = [random.randint(0, 1) for _ in range(chromosome_length)]
population.append(chromosome)
return population
# 计算适应度值
def calculate_fitness(chromosome):
fitness = sum(chromosome)
return fitness
# 选择操作
def selection(population):
population_fitness = []
for chromosome in population:
fitness = calculate_fitness(chromosome)
population_fitness.append((chromosome, fitness))
population_fitness.sort(key=lambda x: x[1], reverse=True)
selected_population = [x[0] for x in population_fitness[:int(population_size * 0.3)]]
return selected_population
# 交叉操作
def crossover(population):
offspring = []
for _ in range(population_size - len(population)):
parent1 = random.choice(population)
parent2 = random.choice(population)
crossover_point = random.randint(1, chromosome_length - 1)
child = parent1[:crossover_point] + parent2[crossover_point:]
offspring.append(child)
return offspring
# 变异操作
def mutation(population):
for chromosome in population:
for i in range(chromosome_length):
if random.random() < mutation_rate:
chromosome[i] = 1 - chromosome[i]
return population
# 主函数
def genetic_algorithm():
population = initialize_population()
for generation in range(max_generations):
selected_population = selection(population)
offspring = crossover(selected_population)
population = mutation(offspring)
population_fitness = [calculate_fitness(chromosome) for chromosome in population]
best_chromosome_index = population_fitness.index(max(population_fitness))
best_chromosome = population[best_chromosome_index]
best_fitness = max(population_fitness)
print("Best Chromosome:", best_chromosome)
print("Best Fitness:", best_fitness)
if __name__ == "__main__":
genetic_algorithm()
```
以上代码实现了一个简单的遗传算法,其中定义了种群大小、染色体长度、迭代次数和突变概率等参数。主要过程包括初始化种群、计算适应度值、选择、交叉和变异等操作,并通过迭代进化获得最优染色体和适应度值。这里的适应度函数是染色体中1的个数。最终输出最优染色体和最优适应度值。
阅读全文
相关推荐
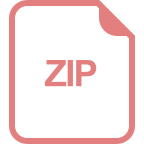
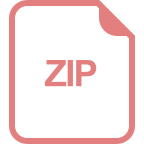
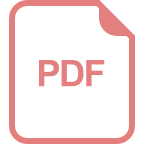
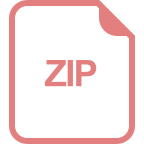
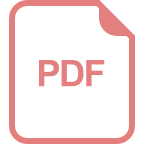