react中useState定义的setData(prevList)除了这个参数还有哪个参数
时间: 2023-12-10 08:37:14 浏览: 28
`setData` 函数是 `useState` 的返回值之一,它只有一个参数,即要设置的新状态值。在使用 `setData` 时,通常需要使用之前的状态值进行计算,以确保状态的正确性。因此,`setData` 函数的参数通常是一个回调函数,该回调函数接收一个参数,即之前的状态值,例如:
```javascript
const [list, setList] = useState([]);
function addItem(item) {
setList(prevList => [...prevList, item]);
}
// 使用
addItem("苹果");
addItem("香蕉");
console.log(list); // ["苹果", "香蕉"]
```
在上面的例子中,`setData` 的参数是一个回调函数 `(prevList) => [...prevList, item]`,其中 `prevList` 是之前的状态值。这个回调函数返回一个新的数组,该数组是之前的状态值 `prevList` 加上新的元素 `item`。因此,`setData` 的作用就是将新的状态值(即新的数组)保存到 `list` 变量中。
相关问题
react React.useState设置参数类型为数字怎么写
在 React 中,可以使用 TypeScript 或 PropTypes 为组件中的 props 和 state 设置类型。对于使用 useState Hook 设置数字类型的 state,可以这样写:
1. TypeScript:
```typescript
import React, { useState } from "react";
interface Props {}
const ExampleComponent: React.FC<Props> = () => {
const [count, setCount] = useState<number>(0);
const handleIncrement = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={handleIncrement}>Increment</button>
</div>
);
};
export default ExampleComponent;
```
2. PropTypes:
```javascript
import React, { useState } from "react";
import PropTypes from "prop-types";
const ExampleComponent = (props) => {
const [count, setCount] = useState(0);
const handleIncrement = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={handleIncrement}>Increment</button>
</div>
);
};
ExampleComponent.propTypes = {
// other PropTypes
count: PropTypes.number,
};
export default ExampleComponent;
```
react 中 useState放在哪里
useState 是 React 中的一个 Hook,它可以用于在函数组件中定义和更新组件的状态变量。useState 应该放在函数组件的顶部,通常是在函数组件的第一行或第二行位置,以确保在函数组件的每次渲染周期中都会被调用。例如:
```
import React, { useState } from 'react';
function Example() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
```
在这个例子中,useState 用于定义一个名为 "count" 的状态变量和一个名为 "setCount" 的用于更新 "count" 变量的函数。在函数组件的返回值中,"count" 变量的值会被显示在页面上,并且在点击按钮时,"setCount" 函数会被调用以更新 "count" 变量的值。
相关推荐
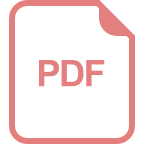
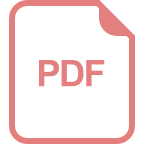
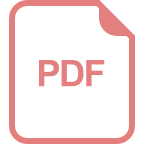












