在已实现下列功能的基础上写一段简单代码实现宽度优先搜索算法(1)棋盘状态的可视化;(2)棋盘状态判断(判断是否为目标状态);(3)规则允许的棋盘操作(下一步棋的算子)。
时间: 2024-01-01 12:04:29 浏览: 30
好的,我明白了。以下是一个简单的宽度优先搜索算法的代码,实现了对一个3x3的棋盘进行状态的搜索和判断:
```python
import numpy as np
from queue import Queue
# 定义初始状态和目标状态
init_state = np.array([[2, 8, 3], [1, 6, 4], [7, 0, 5]])
goal_state = np.array([[1, 2, 3], [8, 0, 4], [7, 6, 5]])
# 定义可行的棋盘操作函数
def get_next_states(state):
next_states = []
zero_pos = np.argwhere(state == 0)[0]
for move in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
next_pos = zero_pos + np.array(move)
if 0 <= next_pos[0] < 3 and 0 <= next_pos[1] < 3:
next_state = state.copy()
next_state[zero_pos[0], zero_pos[1]] = state[next_pos[0], next_pos[1]]
next_state[next_pos[0], next_pos[1]] = 0
next_states.append(next_state)
return next_states
# 定义判断是否为目标状态的函数
def is_goal_state(state):
return np.array_equal(state, goal_state)
# 定义可视化函数
def visualize_state(state):
for row in state:
print(row)
# 宽度优先搜索算法
def bfs(init_state):
visited = set()
q = Queue()
q.put((init_state, []))
while not q.empty():
state, path = q.get()
if is_goal_state(state):
return path
for next_state in get_next_states(state):
if tuple(map(tuple, next_state)) not in visited:
visited.add(tuple(map(tuple, next_state)))
q.put((next_state, path + [next_state]))
# 运行算法并输出结果
path = bfs(init_state)
if path:
print("The solution is found with {} steps:".format(len(path)))
for state in path:
visualize_state(state)
print()
else:
print("The solution is not found.")
```
代码中,我们使用了numpy库来处理棋盘状态,使用queue库来实现广度优先搜索算法。可行的棋盘操作函数`get_next_states`将当前状态下一步所有可能的状态返回,判断是否为目标状态的函数`is_goal_state`判断当前状态是否为目标状态。可视化函数`visualize_state`将当前状态以棋盘形式展示出来。最后,我们使用广度优先搜索算法`bfs`来搜索出从初始状态到目标状态的路径,并输出结果。
需要注意的是,我们在搜索过程中需要对每个状态进行去重,避免重复搜索。这里我们使用了一个集合`visited`来存储已经访问过的状态。
相关推荐
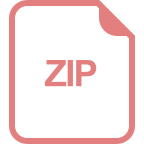
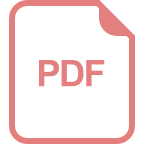














