python二维数组行遍历
时间: 2023-06-23 18:10:09 浏览: 156
在 Python 中,可以使用循环来遍历二维数组的每一行。具体实现可以使用以下代码:
```python
# 定义一个二维数组
arr = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# 遍历二维数组的每一行
for row in arr:
print(row)
```
输出结果为:
```
[1, 2, 3]
[4, 5, 6]
[7, 8, 9]
```
在上面的代码中,我们使用 `for` 循环遍历了二维数组 `arr` 的每一行,并将每一行输出到控制台。如果你需要对每一行进行操作,只需要在循环中添加相应的代码即可。
相关问题
python二维数组回形遍历
以下是Python实现二维数组回形遍历的代码示例:
```python
def spiralOrder(matrix):
if not matrix:
return []
m, n = len(matrix), len(matrix[0])
left, right, top, bottom = 0, n - 1, 0, m - 1
res = []
while left <= right and top <= bottom:
for i in range(left, right + 1):
res.append(matrix[top][i])
top += 1
for i in range(top, bottom + 1):
res.append(matrix[i][right])
right -= 1
if top <= bottom:
for i in range(right, left - 1, -1):
res.append(matrix[bottom][i])
bottom -= 1
if left <= right:
for i in range(bottom, top - 1, -1):
res.append(matrix[i][left])
left += 1
return res
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
result = spiralOrder(matrix)
print(result) # 输出:[1, 2, 3, 6, 9, 8, 7, 4, 5]
```
python二维数组遍历
在Python中,可以使用嵌套的for循环来遍历二维数组。例如,假设有一个3行4列的二维数组arr:
``` python
arr = [[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12]]
```
那么可以使用以下代码遍历这个二维数组中的所有元素:
``` python
for i in range(len(arr)):
for j in range(len(arr[i])):
print(arr[i][j])
```
其中,外层的for循环用来遍历每一行,内层的for循环用来遍历每一行中的元素。在这个例子中,输出的结果将是:
```
1
2
3
4
5
6
7
8
9
10
11
12
```
当然,也可以使用更简单的方式来遍历二维数组,例如使用列表推导式:
``` python
[element for row in arr for element in row]
```
这行代码的含义是先遍历每一行,再遍历每一行中的元素,最终将所有元素放入一个新的列表中。这个新列表的元素顺序与原二维数组中的元素顺序相同。
阅读全文
相关推荐
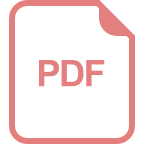
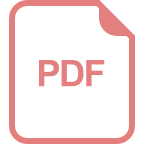













