结合二叉树遍历算法,在下列二叉树遍历的应用中任选一题完成。 1. 统计二叉树中叶子结点的个数;用c语言实现
时间: 2023-06-19 22:03:19 浏览: 47
下面是统计二叉树中叶子结点个数的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *left;
struct node *right;
} Node;
// 创建二叉树
Node* createTree() {
Node *root = (Node *) malloc(sizeof(Node));
root->data = 1;
root->left = (Node *) malloc(sizeof(Node));
root->left->data = 2;
root->left->left = (Node *) malloc(sizeof(Node));
root->left->left->data = 4;
root->left->left->left = NULL;
root->left->left->right = NULL;
root->left->right = (Node *) malloc(sizeof(Node));
root->left->right->data = 5;
root->left->right->left = NULL;
root->left->right->right = NULL;
root->right = (Node *) malloc(sizeof(Node));
root->right->data = 3;
root->right->left = (Node *) malloc(sizeof(Node));
root->right->left->data = 6;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right = (Node *) malloc(sizeof(Node));
root->right->right->data = 7;
root->right->right->left = NULL;
root->right->right->right = NULL;
return root;
}
// 统计二叉树中叶子结点个数
int countLeaves(Node* root) {
if (root == NULL)
return 0;
if (root->left == NULL && root->right == NULL)
return 1;
return countLeaves(root->left) + countLeaves(root->right);
}
int main() {
Node *root = createTree();
int count = countLeaves(root);
printf("Leaves count: %d\n", count);
return 0;
}
```
输出结果为:
```
Leaves count: 4
```
相关推荐
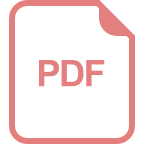












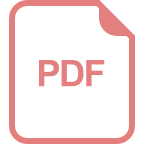
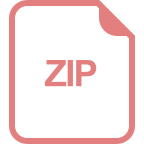