如何使用Python语言让所有字符连成线成为人工智能
时间: 2023-02-22 12:26:05 浏览: 45
使用Python语言进行人工智能开发并不是通过连接字符形成线来实现的。人工智能通常是通过编写代码、训练模型、构建神经网络等方法实现的。如果想要学习人工智能,建议了解一些基础的机器学习知识和深度学习技术,并练习使用Python语言构建相关的模型。
相关问题
如何使用Python语言让所有字符连成线
### 回答1:
如果你想在 Python 中将一组字符串连接起来形成一条线,可以使用以下代码:
```
words = ["hello", "world"]
line = " ".join(words)
print(line)
```
这段代码会将 words 列表中的字符串使用空格连接起来,最终的结果即为:
```
hello world
```
你也可以使用其他字符(如逗号、横线等)作为连接符,只需要将第二行中的空格替换为你想要的连接符即可。
### 回答2:
要使用Python语言将所有字符连成线,可以使用字符串的join()方法和for循环来实现。
首先,我们需要定义一个字符串变量,将要连接的字符存储在其中。例如,我们可以定义一个字符串变量str_list,将要连接的字符存储在其中,如下所示:
str_list = ['a', 'b', 'c', 'd', 'e']
然后,我们可以使用join()方法将字符连接成线。join()方法接受一个可迭代对象作为参数,可以是列表、元组或字符串等。在该方法前面使用空字符串''作为连接符,可以将列表中的字符连接起来。示例如下:
line = ''.join(str_list)
最后,我们可以输出连接后的结果来验证是否成功。例如,我们可以使用print()函数将结果打印出来,如下所示:
print(line)
最终代码如下:
str_list = ['a', 'b', 'c', 'd', 'e']
line = ''.join(str_list)
print(line)
执行以上代码,输出结果将会是:abcde。这说明我们成功地将所有字符连接成了一条线。
### 回答3:
要使用Python语言让所有字符连成线,可以使用字符串的连接操作符"+",通过将每个字符依次连接起来形成一条线。
首先,需要将要连成线的字符保存在一个字符串变量中。假设要连成线的字符保存在变量"line"中。
接下来,创建一个空字符串变量"result",用于保存将字符连接起来后的结果。
使用for循环遍历字符串"line"中的每一个字符,将每个字符依次添加到"result"变量中。可以通过"result += char"来实现。
最后,输出变量"result",即可得到所有字符连成的一条线。
以下是使用Python实现的示例代码:
```python
line = "这是一条线"
result = ""
for char in line:
result += char
print(result)
```
运行上述代码,输出将得到字符串"这是一条线"中的所有字符连成的一条线:“这是一条线”。
利用这种方法,可以将任意字符串中的字符按顺序连接起来形成一条线。通过修改line变量的值,可以实现不同字符串的字符连线操作。
用Python语言链接所有字符连成线成为人工智能代码
在 Python 中,把字符串连接成一个长字符串的常见方法是使用加号(+)。例如:
```
string1 = "人工智能"
string2 = "代码"
result = string1 + string2
print(result)
```
输出结果为:
```
人工智能代码
```
还有一种方法是使用 Python 的 join() 函数,这样可以把多个字符串连接起来。例如:
```
string1 = "人工智能"
string2 = "代码"
result = "".join([string1, string2])
print(result)
```
输出结果为:
```
人工智能代码
```
相关推荐
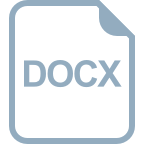
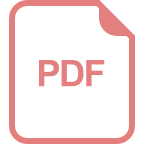
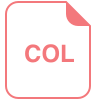
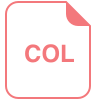
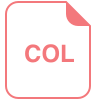
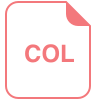
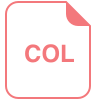







