c++ string.find()存在多个符合
时间: 2024-02-28 22:29:45 浏览: 40
`string::find()` 方法返回第一个匹配子字符串的位置,如果没有找到则返回 `string::npos`。如果你想查找字符串中所有符合条件的子字符串,可以使用一个循环和 `string::find()` 方法来不断查找。每次找到匹配子字符串后,将其位置加上子字符串长度,然后继续查找下一个匹配子字符串,直到找不到为止。
下面是一个示例代码,可以查找字符串中所有的 `hello` 子字符串:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "hello world, hello c++, hello python";
std::string sub_str = "hello";
std::size_t pos = str.find(sub_str);
while (pos != std::string::npos) {
std::cout << "Found at position " << pos << std::endl;
pos = str.find(sub_str, pos + sub_str.size());
}
return 0;
}
```
输出结果为:
```
Found at position 0
Found at position 13
Found at position 22
```
相关问题
string.find 函数时间复杂度c++
string.find函数是C++中用于在字符串中查找子串的函数。它的时间复杂度为O(n*m),其中n是原字符串的长度,m是要查找的子串的长度。
具体来说,string.find函数会从原字符串的起始位置开始逐个字符地与子串进行比较,直到找到匹配的子串或者遍历完整个原字符串。在最坏情况下,需要比较的次数为n-m+1次,因此时间复杂度为O(n*m)。
需要注意的是,如果要多次查找同一个子串,可以考虑使用KMP算法等更高效的字符串匹配算法来提高查找效率。
c++中string 的find
在C++中,string类有一个成员函数find(),它用于查找字符串中的子串。find()函数有多个重载版本,可以根据不同的参数进行使用。以下是其中几个常用的重载版本:
1. `size_t find(const string& str, size_t pos = 0) const`
这个版本的find()函数会在当前字符串中从指定位置pos开始查找子串str。如果找到,返回子串的起始位置;如果没找到,返回string::npos。
2. `size_t find(const char* s, size_t pos = 0) const`
这个版本的find()函数会在当前字符串中从指定位置pos开始查找C风格字符串s。如果找到,返回子串的起始位置;如果没找到,返回string::npos。
3. `size_t find(char c, size_t pos = 0) const`
这个版本的find()函数会在当前字符串中从指定位置pos开始查找字符c。如果找到,返回字符的位置;如果没找到,返回string::npos。
下面是一个示例代码,演示如何使用find()函数查找子串:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::size_t found = str.find("World");
if (found != std::string::npos) {
std::cout << "Substring found at position: " << found << std::endl;
} else {
std::cout << "Substring not found" << std::endl;
}
return 0;
}
```
输出结果为:
```
Substring found at position: 7
```
以上是C++中string的find()函数的简单介绍,希望对你有所帮助!如果有更多问题,请随时提问。
相关推荐
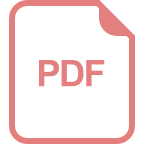
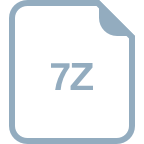
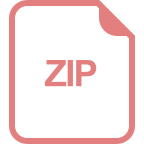












