C++ 将数据写成压缩文件
时间: 2024-02-21 22:57:30 浏览: 115
要将数据写成压缩文件,需要使用压缩库。C++中常用的压缩库有zlib和gzip。
下面是使用gzip压缩库将数据写成压缩文件的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <zlib.h>
using namespace std;
int main()
{
string data = "Hello, world! This is some data to be compressed.";
// 打开文件
ofstream file("data.txt");
if (!file.is_open()) {
cout << "Error: Failed to open file." << endl;
return 1;
}
// 写入数据
file << data;
// 关闭文件
file.close();
// 打开压缩文件
gzFile compressed_file = gzopen("compressed_file.gz", "wb");
if (compressed_file == NULL) {
cout << "Error: Failed to open compressed file." << endl;
return 1;
}
// 打开原始数据文件
ifstream data_file("data.txt", ios::binary);
if (!data_file.is_open()) {
cout << "Error: Failed to open data file." << endl;
return 1;
}
// 压缩数据并写入文件
char buffer[1024];
int num_read = 0;
while ((num_read = data_file.read(buffer, sizeof(buffer)).gcount()) > 0) {
if (gzwrite(compressed_file, buffer, num_read) != num_read) {
cout << "Error: Failed to write compressed data." << endl;
return 1;
}
}
// 关闭文件
gzclose(compressed_file);
data_file.close();
// 删除原始数据文件
remove("data.txt");
return 0;
}
```
在上面的代码中,我们首先将数据写入到一个普通的文本文件`data.txt`中,然后打开压缩文件,打开原始数据文件,从原始数据文件中读取数据,压缩并写入到压缩文件中。最后删除原始数据文件。需要注意的是,压缩文件的后缀名应该是`.gz`。
阅读全文
相关推荐
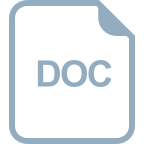
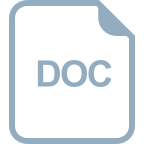
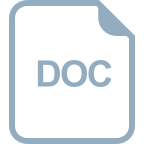

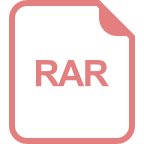
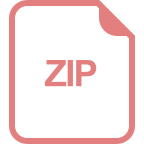
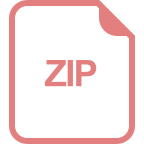
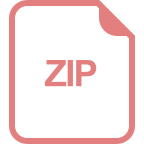
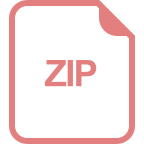
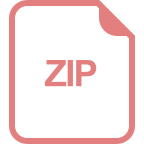
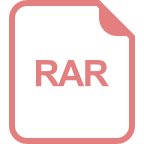
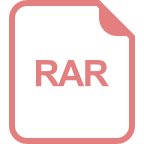
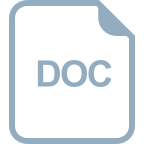
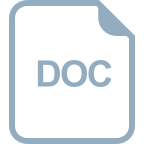
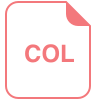



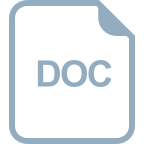