如何利用结构体在一个for循环中做多条曲线的二维图
时间: 2024-05-13 12:20:50 浏览: 66
可以先定义一个包含多条曲线信息的结构体,例如:
```
typedef struct {
double* x; // x坐标数组
double* y; // y坐标数组
int n; // 数据点数目
char* color; // 曲线颜色
} curve;
```
然后在主函数中定义多个曲线的结构体,每个结构体包含一条曲线的信息,例如:
```
curve curve1 = {x1, y1, n1, "r"};
curve curve2 = {x2, y2, n2, "b"};
curve curve3 = {x3, y3, n3, "g"};
...
```
其中,x1,y1,n1是第一条曲线的x坐标数组、y坐标数组和数据点数目,"r"表示该曲线的颜色为红色。
接着,在一个for循环中,依次画出每条曲线,可以使用for循环遍历所有的曲线结构体,对于每个结构体,使用Matplotlib库中的plot函数画出对应的曲线,例如:
```
for (int i = 0; i < num_curves; i++) {
curve cur = curves[i];
plt::plot(cur.x, cur.y, cur.color);
}
```
其中,num_curves表示曲线的数量,curves是一个包含所有曲线结构体的数组。
最后,使用Matplotlib库中的show函数显示出所有曲线的图像,例如:
```
plt::show();
```
完整示例代码如下:
```
#include <iostream>
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
typedef struct {
double* x;
double* y;
int n;
char* color;
} curve;
int main() {
// 定义多条曲线的结构体
double x1[] = {0, 1, 2, 3, 4};
double y1[] = {1, 2, 3, 4, 5};
curve curve1 = {x1, y1, 5, "r"};
double x2[] = {0, 1, 2, 3, 4};
double y2[] = {2, 3, 4, 5, 6};
curve curve2 = {x2, y2, 5, "b"};
double x3[] = {0, 1, 2, 3, 4};
double y3[] = {3, 4, 5, 6, 7};
curve curve3 = {x3, y3, 5, "g"};
// 遍历所有曲线结构体,依次画出每条曲线
curve curves[] = {curve1, curve2, curve3};
int num_curves = 3;
for (int i = 0; i < num_curves; i++) {
curve cur = curves[i];
plt::plot(cur.x, cur.y, cur.color);
}
// 显示图像
plt::show();
return 0;
}
```
阅读全文
相关推荐


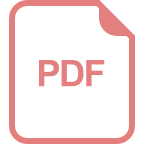














